AWS Cloud Operations Blog
How to automate AWS Support API with Amazon EventBridge
The practice of Operational Integration defines how one organization’s people, processes, and tools integrate with the people, processes, and tools of another organization. When certain activities occur within one organization, it may trigger an automated or manual response in another. For example, it’s common for AWS customers to operationally integrate with AWS Support or AWS Managed Services whenever they need help from AWS.
AWS Support recently announced Amazon EventBridge support for the AWS Support API. In the past, customers who wanted to automate a custom response to Support Case activity needed to poll AWS APIs to detect when cases had been created or updated. Now customers can use Amazon EventBridge to initiate Event-Driven workflows when activities take place within their AWS account. EventBridge can automatically detect AWS Support activity, as well as initiate an automated response.
This post will detail how you can embrace an event-driven architecture for common automation scenarios, and interact programmatically with the Support API.
Solution architecture
This solution is deployed as an AWS CloudFormation stack that deploys the following resources into your AWS account:
- An EventBridge rule to initiate automated actions when AWS Support Cases are created or updated.
- An Amazon Simple Notification Service (Amazon SNS) topic to which the EventBridge rule will publish when support activity has taken place.
- An AWS Lambda function that publishes notifications to an SNS topic.
- An SNS topic that interested parties can subscribe to when they want to be notified of support activity taking place in their account.
- A Lambda function that initiates a Slack message to notify a Slack Channel that support activity has taken place.
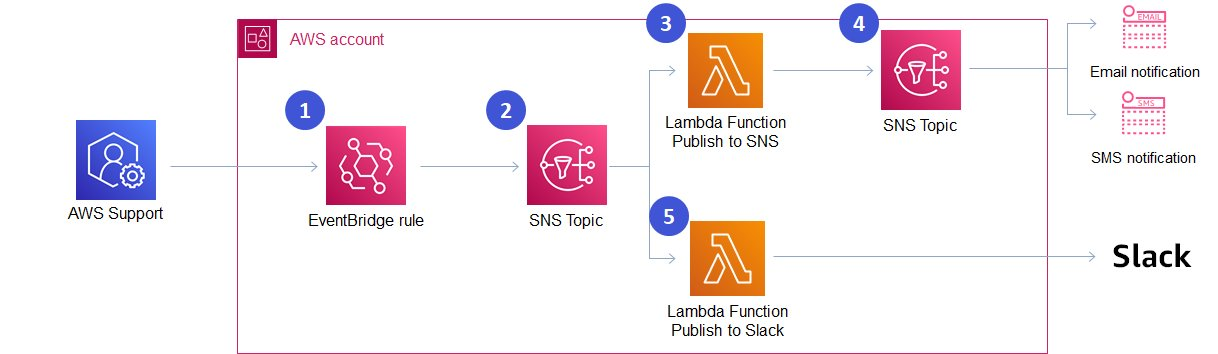
A Solution Architecture diagram depicting an automated process that is triggered whenever AWS Support activity takes place.
The solution works as follows:
- A Support Case is created or updated inside of your account.
- An Event is put on the EventBridge event bus, and this event is evaluated by EventBridge to determine if there is a matching EventBridge rule.
- An EventBridge rule is triggered when the event matches the following pattern:
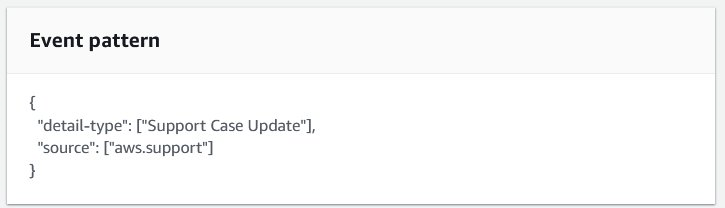
A screenshot from the AWS Console depicting an EventBridge Pattern that matches AWS
- The EventBridge rule publishes the event to an SNS Topic.
- The SNS Topic triggers two Lambda functions, passing a copy of the event to both functions:
-
- The first Lambda function evaluates the event, and based on whether the event is a ‘Create’ or ‘Update’ event, publishes a user-friendly message to an SNS topic. Users who are subscribed to this SNS topic will receive notifications (for example, Email or SMS)
- The second Lambda function evaluates the event and makes an API call to the Slack Webhook API. Assuming that Slack is configured to receive these events, a message will be posted to a Slack Channel to notify members of the channel of AWS Support activity.
Considerations for production use
The following considerations should be taken into account for use in production:
Encryption – It’s best practice to use encryption everywhere. In the sample code provided with this post, the SNS topics aren’t encrypted. When using this solution in production, you should encrypt these topics using AWS Key Management Service (AWS KMS).
At least once delivery – Amazon SNS provides “at least once” delivery of messages, which means that there’s a chance you might receive multiple notifications. This may not be critical to your organization when integrating with Email or Slack. However, it may present challenges in more complex automations. Consider mechanisms to provide exactly-once delivery based on your use-case.
Reserved concurrency – If the AWS account in which this solution is deployed also uses other AWS Lambda functions, then there’s a risk that other Lambda Functions may consume all of the available account capacity, and prevent the Lambda functions in this solution from running. You may need to consider reserved concurrency if this is applicable to your use case.
Log retention – The sample code provided with this post has a hard-coded Amazon CloudWatch Logs retention period of seven days. Customers should consider their own data storage retention policies when using it in production.
Procedures
Prerequisites
The following prerequisites are crucial:
- An AWS Account with AWS Command Line Interface (AWS CLI) configured.
- The AWS account must be enrolled in Business Support, Enterprise On-Ramp, or Enterprise Support to access the AWS Support API.
- A Slack channel that is configured for advanced workflows using webhooks based on the documented steps on this page. This post also summarizes those steps in the following section.
- Git to download the sample file source code.
Step 1: Configure Slack to receive notifications about AWS Support activity
A slack webhook is an HTTPS URL that will receive an HTTPS Request whenever Support Activity occurs inside of the AWS Account. When the Webhook receives a properly formatted request, it will pass on the information in the form of a message to a slack channel. Slack’s documentation describes how to create advanced workflows using webhooks. However, the key steps are covered in the following section.
First, if you don’t have a slack channel in which you would like AWS Support activity to be published, then create a new Slack channel.
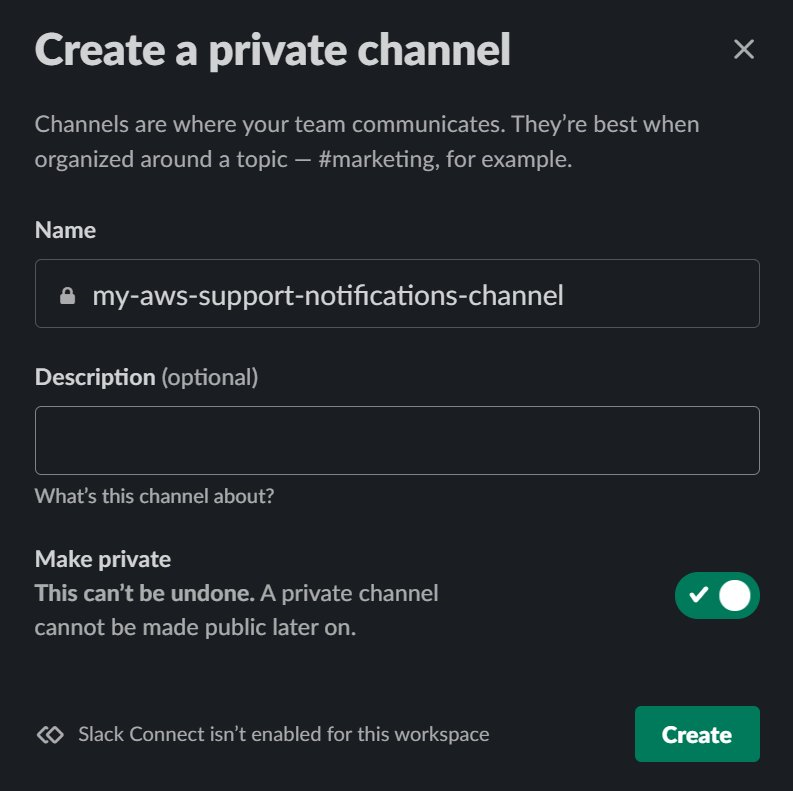
From the channel in which you would like AWS Support activity to be published, open the Slack Workflow Builder.
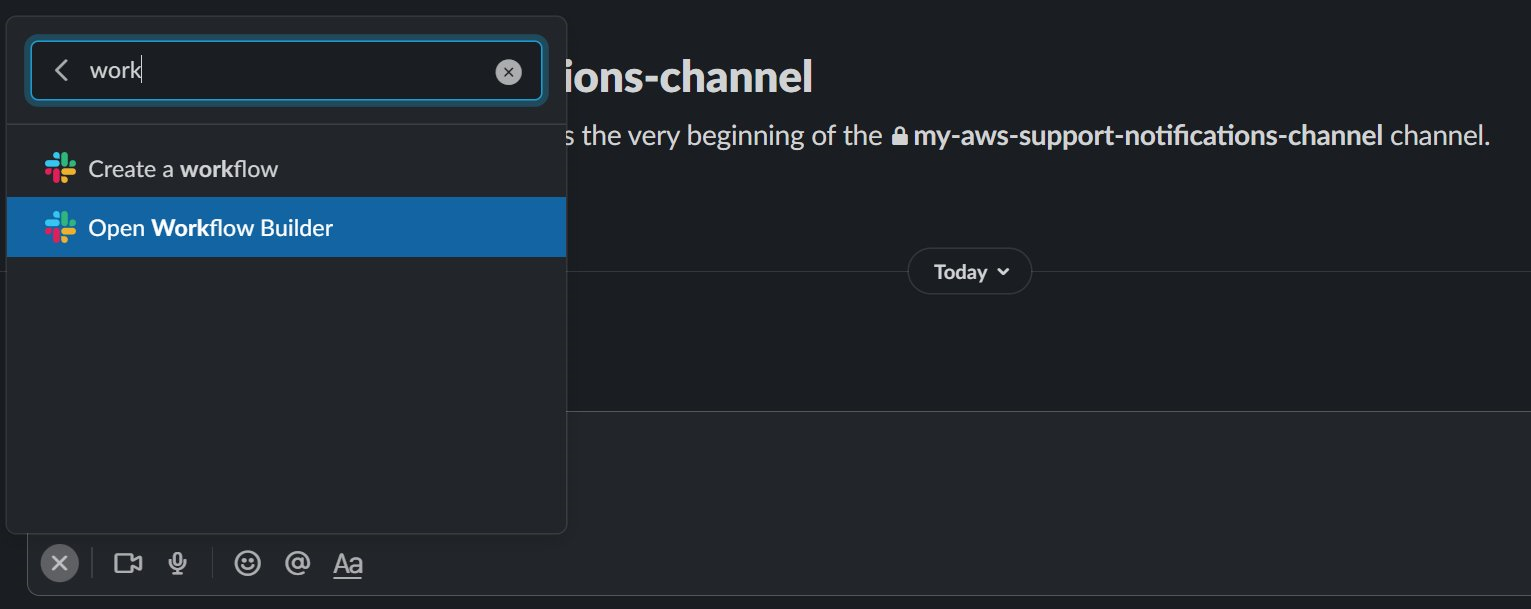
Select the “Create” button, and give your workflow a name.
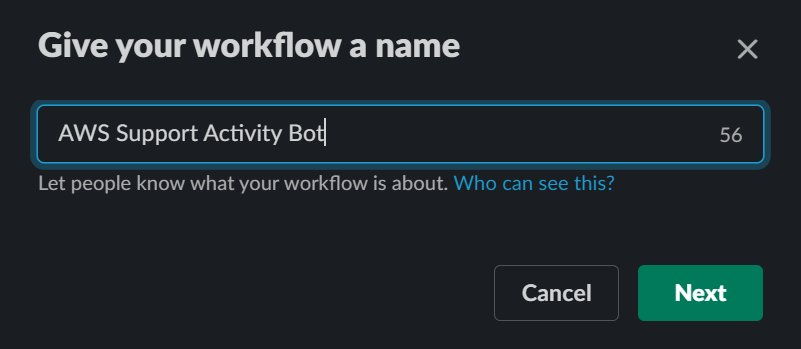
Select the “Webhook” option to start this workflow.
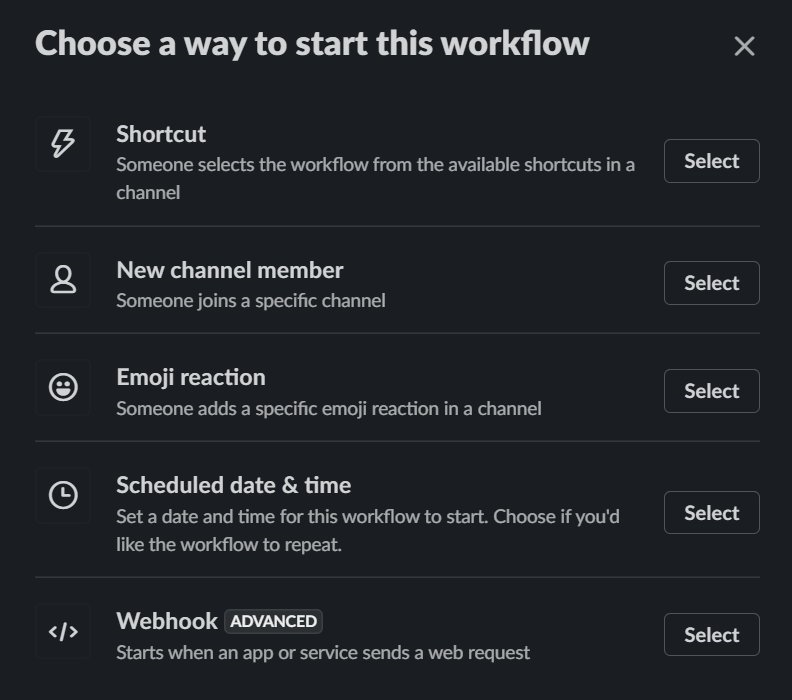
Create 3 variables (caseId, caseUrl, updateDetails), each with a Data Type of “Text”. The variables are case sensitive.
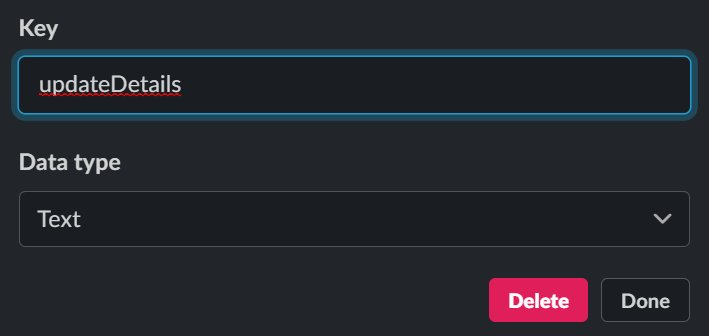
Next, add a workflow step to “Send a Message” when the webhook receives a message.
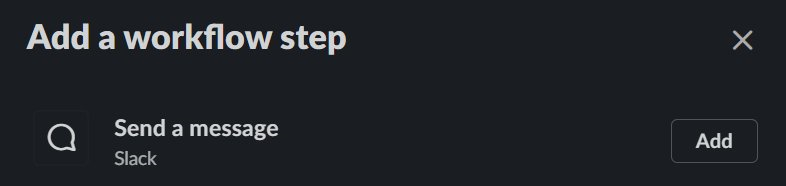
Select a slack channel in which you would like to publish AWS Support activity. You can customize the message that will be sent to the channel according to your own requirements, and insert the three variables created earlier (caseId, caseUrl, and updateDetails).
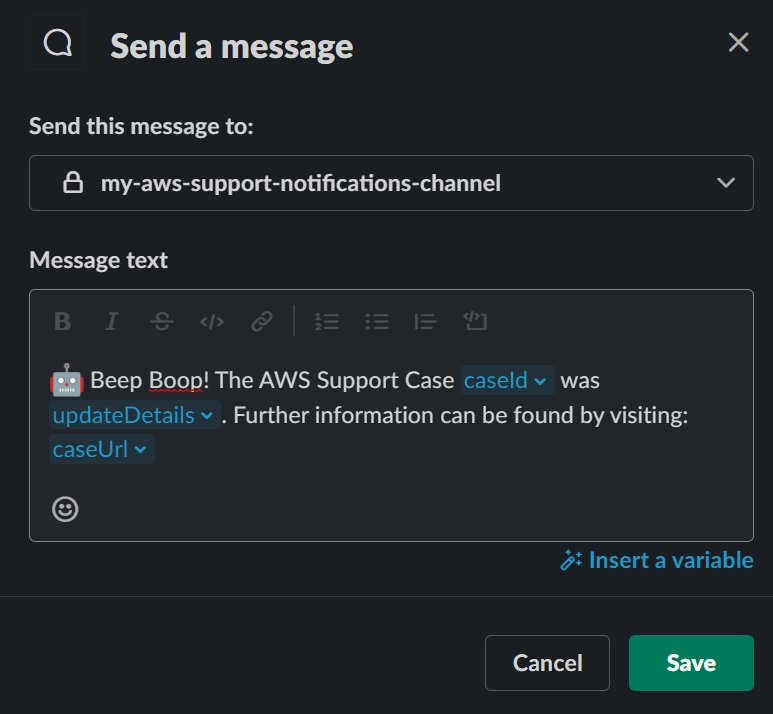
Select the “Publish” option to create the Webhook. After publishing, copy the Slack Webhook URL, which will be similar to: https://hooks.slack.com/workflows/
Step 2: Deploy this solution using the AWS CLI into the us-east-1 region
The AWS Support API is accessible via us-east-1 endpoint. Therefore, this solution must be deployed in the us-east-1 region. Update the SlackWebhookURL parameter below with the Webhook URL created during the previous step.
Step 3: Subscribe an email address to the SNS topic that provides case update notifications
After your CloudFormation stack has been deployed, navigate to the Amazon SNS console, and select the topic containing the name “EndUserSubscriptionToipic”.
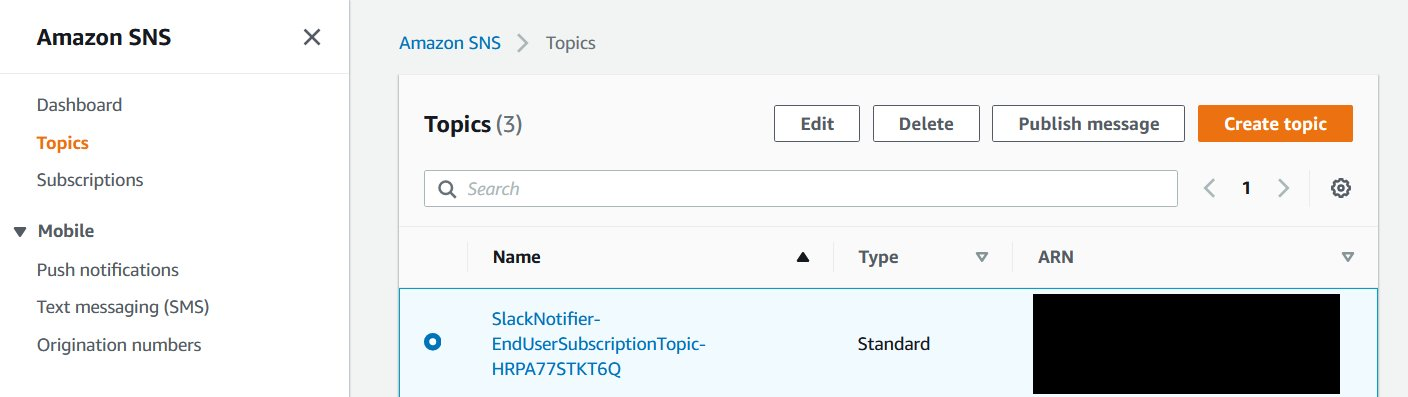
Create a subscription to this topic.
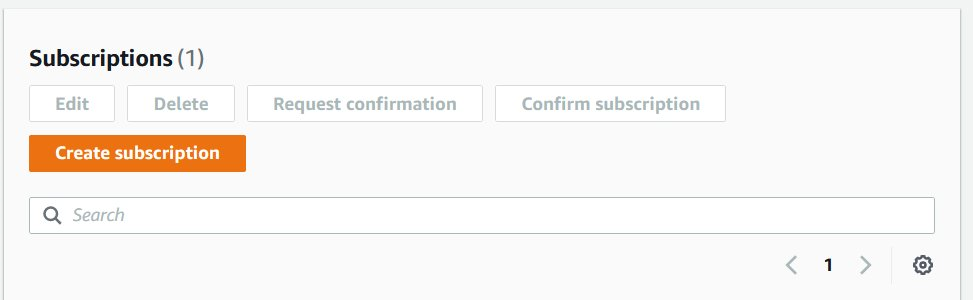
Select the “Email” Protocol, and enter a destination email address that you would like to notify of AWS Support Case activity.
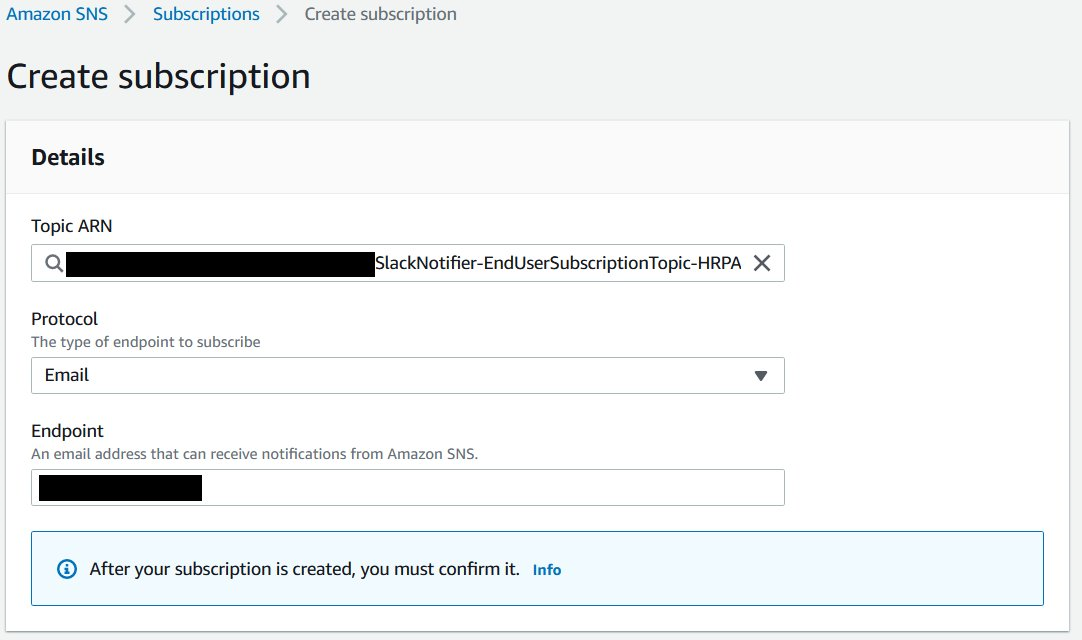
Check your email, as you must confirm the subscription by selecting the provided link before continuing.
Step 4: Create a test AWS Support Case to verify that the solution has been implemented
Using the AWS Console, AWS CLI, or APIs, create a new AWS Support Case. For test cases, use the subject TEST CASE-Please ignore
.
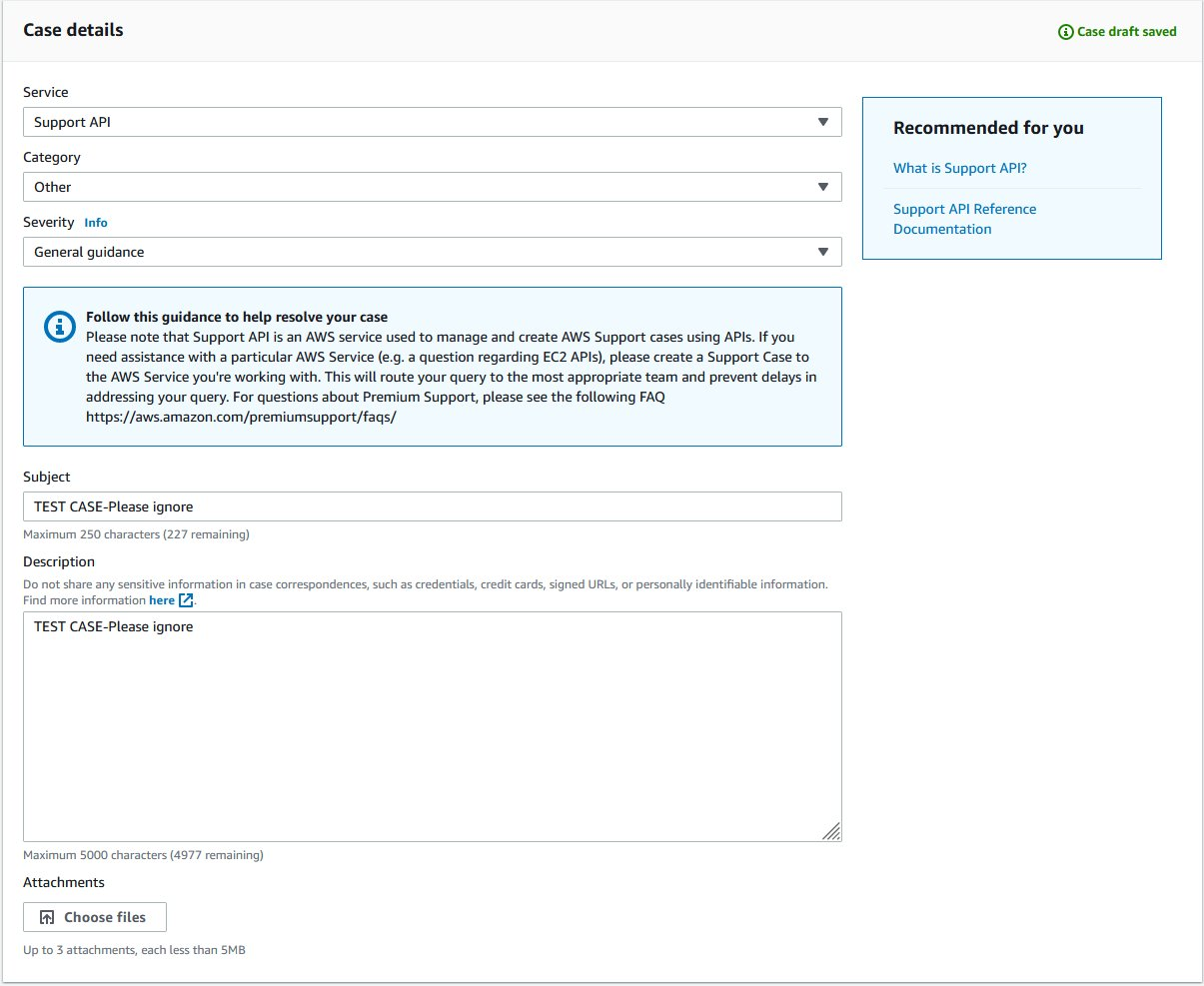
After creating the case, you will receive a notification in your Slack channel that a support case was created.
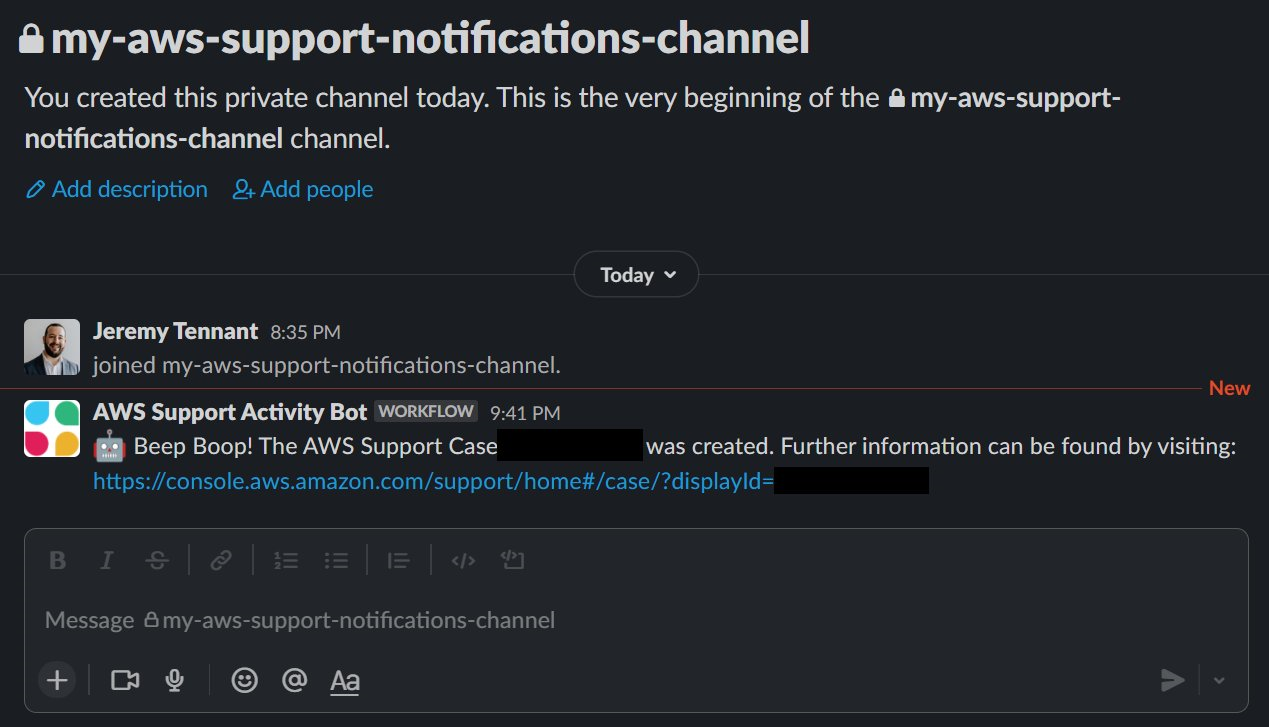
Furthermore, you’ll receive an email notification from the SNS topic to which you subscribed.
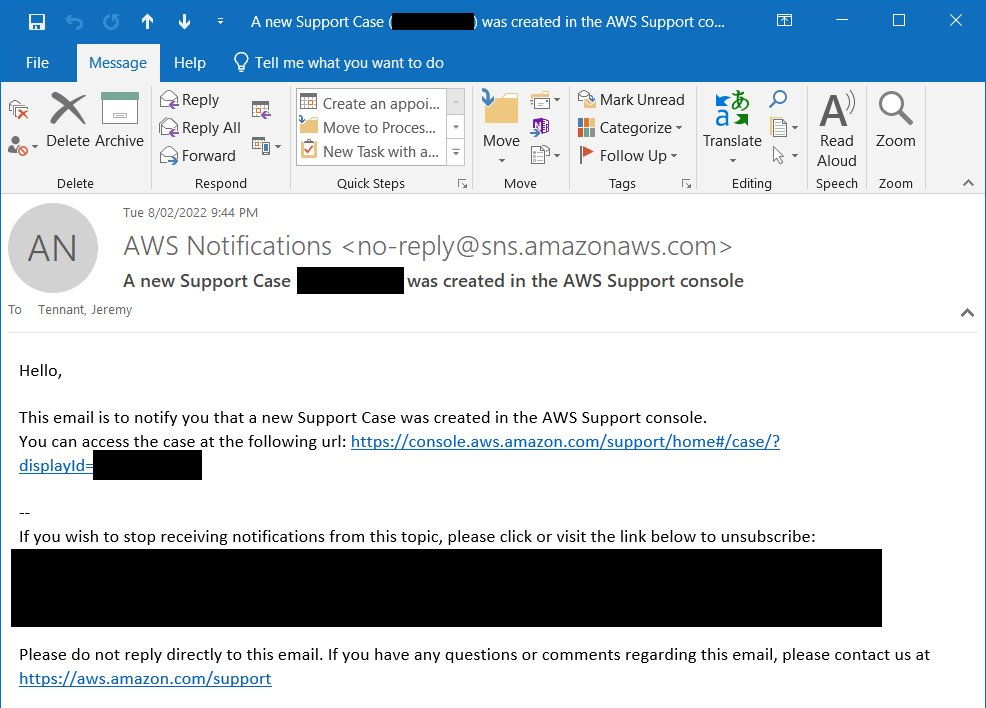
Screenshot from Slack depicting successful message delivery.
Cleanup
When you’re done, you can clean-up the AWS Resources that were deployed by executing the following commands with the AWS CLI:
Conclusion
In this post, I’ve provided a solution that uses EventBridge to trigger one or more Lambda functions whenever support activity occurs within your AWS account. You can also extend this sample code to create a more advanced automation that meets your own custom requirements.
In addition, AWS provides the AWS Service Management Connector for Jira Service Management and AWS Service Management Connector for ServiceNow for customers who would prefer a prescriptive solution to integrate with AWS Support and AWS Managed Services.
Happy building!
Author: