Introduction
A MicroSaaS, short for Micro Software as a Service, is a type of software business model that focuses on providing highly specialized and targeted solutions to meet specific needs of a niche market. Unlike traditional SaaS companies that aim for a broader customer base, MicroSaaS companies develop and offer compact, focused applications designed to address a particular problem or serve a specific customer segment.
These applications are often characterized by their simplicity, ease of use, and efficiency in solving a particular pain point. MicroSaaS is particularly attractive for teams looking to generate monthly recurring revenue (MRR) in the range of $5,000 to $10,000, as it allows them to achieve profitability and sustainability by catering to a specific customer base with a highly tailored product.
Typically composed of 1-5 members, these groups possess diverse skill sets that span the development stack. Recent observations indicate a growing trend among individuals in this community, where frontend developers are increasingly displaying proficiency in backend development.
The audience that falls in this community constantly seek ways to optimize their workflows and create robust, scalable applications that leave their customers delighted. While various frontend frameworks contribute to this objective, some notable ones include React/Next.js, Stripe, TailwindCSS, and TypeScript.
Nonetheless, the backend continues to be a realm plagued by decision paralysis, and understandably so. Crucial elements such as authentication and authorization (authN/authZ), file storage, database flexibility, and APIs form the backbone of any practical application but present obstacles when transitioning from existing solutions. As a result, developers often gravitate towards the simplest setup to commence their development journey.
This guide introduction and the following guides in this 'front-end developer guide for building on AWS' series intend to answer why AWS, specifically a core selection of tools and services, increases full stack velocity, and how this benefit affects end users as an application scales.
Here's a quick overview of all the guides in this series:
- (This guide): Why your next Micro-SaaS should be powered by AWS
- Getting started: How to install the AWS CLI and configure a CDK TypeScript project
- AWS CDK for Front-End Developers: The Ultimate Guide to Get You Started
- AWS CDK for Front-End Developers: Databases and Serverless Functions
- The AWS CDK Guide to Authentication & Authorization for Front-End Developers
- AWS CDK for Front-End Developers: Amazon S3 and CloudFront
- AWS CDK for Front-End Developers: Building Modern APIs with AWS AppSync
- AWS CDK for Front-End Developers: Multi-Stage Deploys with Github Actions
Reasons for a Change in Technical Overhead
The alternative to building backend infrastructure on AWS is often to off-load to a third-party provider. In a bit of a drastic sense, this could mean for each part of your backend infrastructure (auth, database, file storage, etc), a different third-party provider is selected. Not only does this create multiple points of failures that don’t seamlessly integrate with one another, but a variable cost factor that is often hidden by attractive getting started options.
If not already familiar with AWS, there is a learning curve. However, this learning curve comes with the benefit of owning the data, serverless pricing models that scale, and more control over how services can be fine-tuned. Each service still has a point of failure; however, the services operate closer together thus making error handling more unified across the stack.
That isn't to say low-code tools don't have their place or benefit. Rather, it's about retaining ownership, and thus having more control over what is happening in your application. Recall that AWS is an ecosystem of services. Having services that know how to talk to and integrate with each other is a huge benefit. In the end, low-code tools remain extremely viable (AWS has several), and when considering a 3rd-party tool there is often a "Better-Together" workflow as well.
Reasons for Biasing Towards Managed and Serverless Solutions
It’s certainly fair to say: If I'm not using a service, then don't charge me. Focusing on serverless and managed services offered by AWS enables you to build scalable, cost-effective applications without managing servers. AWS offers a range of serverless services like AWS Lambda, Amazon API Gateway, and Amazon DynamoDB, allowing you to build and deploy your applications quickly, with built-in scalability and cost optimization. As a CTO, indie creator or SaaS founder, cost and durability are likely your biggest factors. This is where a managed service comes into play. Managed means less time provisioning throughput, less code you have to write, and more time focusing on your product and customers.
However, people often think this means more Lambda functions, when it really means fewer Lambda functions. A large benefit of serverless, fully-managed services on AWS is there are often direct integrations available so they can talk to one another. Less Lambda functions mean fewer cold starts. AWS Step Functions, Amazon EventBridge Pipes, and AWS AppSync are great examples of this.
Modern Front-End App Development with Next.js and Tailwind
With almost half of all respondents in the 2022 State of JS survey using Next.js and a 90% retention rate, Next.js has become the staple when it comes to application development.
Embracing modern frontend development means adopting powerful tools like Next.js and Tailwind CSS. Next.js, a popular framework built on top of React, brings numerous benefits, such as built-in TypeScript support, which enables robust type-checking and code autocompletion, and file-based routing for effortless page creation and navigation. With its hybrid rendering, you choose between server-side rendering (SSR), static site generation (SSG), or client-side rendering, depending on your application's needs.
With 46% of the State of CSS survey respondents using Tailwind, and the highest retention rate (79%) of CSS frameworks, Tailwind, then, is a good choice for your default CSS framework when building new applications.
Tailwind CSS is a utility-first CSS framework that streamlines the styling process, making it faster and more efficient. It promotes consistency, maintainability, and readability, allowing you to create responsive, modern designs without writing custom CSS classes. By combining Next.js and Tailwind CSS, you can build high-performance, visually appealing applications while benefiting from an enhanced development experience that keeps you focused on what truly matters: delivering innovative solutions for your customers.
Low Barrier-to-Entry with AWS CDK and TypeScript
//create a database table
const travelTable = new awsDynamodb.Table (scope, 'TravelTable', {
tableName: props.tableName,
removalPolicy: RemovalPolicy.DESTROY,
billingMode: awsDynamodb.BillingMode.PAY_PER_REQUEST,
partitionKey: { name: 'id' , type: awsDynamodb.AttributeType.STRING },
})
// CREATE AN S3 BUCKET WITH CORS FR STORING FILES
const fileStorageBucket = new s3.Bucket (scope, props.bucketName, {
bucketName: props.bucketName
})
While not showcasing every option available, the above image shows everything required to create a database and an S3 bucket that stores files. As a TypeScript enthusiast, you'll love working with the AWS Cloud Development Kit (CDK). Before, creating your AWS Services by clicking through the AWS Console and writing down the steps you took so you didn't forget them next time. When scripting came along, it was either writing your own Bash scripts to use the AWS CLI or writing verbose templates using AWS CloudFormation.
If you haven't used CloudFormation before, just know defining a database like the above was with a YAML file several times longer. With the AWS CDK, you can create, test, and deploy AWS resources a lot easier, streamlining your development process and taking advantage of the full power of AWS services since it exists as a wrapper around CloudFormation.
The AWS CDK ranks 3rd when it comes to building out fully serverless applications, has the highest retention (84%), and has the best positive/negative split.
Simple Front End-Back End Integration with AWS Amplify
AWS Amplify is a suite of services that include a CLI, hosting platform, UI Component Library and more. In particular, the Amplify JavaScript libraries provide a seamless way to connect your frontend and backend, offering pre-built UI components, authentication, and storage solutions.
As an example, developers often have to figure out an easy way to pass a large file from the frontend to some backend storage service. Using the Amplify libraries, files can several GB large and can be sent as a multi-part upload, paused, resumed and canceled.
import {Amplify} from 'aws-amplify'
const upload = await Storage.put ("test.txt", myFile.png);
upload.pause ();
upload.resume ();
upload.cancel (upload);
// or
<FileUploader
maxFileCount={3}
variation="drop"
acceptedFileTypes={['image/*']}
accessLevel="public"
Scalable, Real-Time GraphQL API with AWS AppSync
As a front-end developer, you're probably familiar with the benefits of GraphQL, offering a flexible and efficient way to fetch data. AWS AppSync is a fully managed GraphQL service. You supply it with a schema, give it data sources, and tell it how to connect to the data sources. As more requests come in, the service scales appropriately. If you've ever had Taco Bell delivered to you, watched a show on HBO Max, saw stats on a live Ferrari F1 race, or purchased a ticket from Ticketmaster, then you've already interacted with AWS AppSync.
To resolve the data, you write JavaScript (native TypeScript support coming soon). Note that this isn't in a server, or even a serverless function like Lambda, this is a mapping that tells AppSync how to fetch the data. For example, the following is how a user would grab an item from DynamoDB:
import {util} from 'aws-appsync/utils'
export function request (ctx) {
const getTravePostRequest = {
operation: 'GetItem',
key: util.dynamodb.toMapValues ({id: ctx.args.id }),
}
return getTravePostRequest
}
export function response (ctx) {
return ctx.result
}
To emphasize, when a user on the frontend makes a request, that request goes to AppSync, which then, gets the data directly from DynamoDB. No connections to manage, to extra service to invoke. This is the power of having various resources as part of the same ecosystem of services. To go in the other direction, by specifying the @aws_cognito_user_pools directive on your schema, AppSync will automatically inspect the JWT of the request to make sure the user is authenticated with Amazon Cognito before allowing the request.
type Query {
getItemFromDatabase (id: ID!) : DatabaseItem @aws_cognito_user_pools
}
Indie creators and SaaS founders of all sizes often have changing requirements and unforeseen access patterns to account for. AppSync's flexible schema model means less stress and more innovation.
Conclusion
Adopting AWS for your application development brings a lot of benefits, including a more streamlined and efficient development process. By leveraging the AWS CDK for TypeScript, serverless and managed services, AWS AppSync for GraphQL, and AWS Amplify for frontend-backend integration, you can fully take advantage of your full-stack potential and build innovative, scalable applications.
This was an introduction to this guide series where I'll walk you through building a full-stack application using the tools and services we discussed. I'll show you how to get started, from setting up your AWS environment to deploying your Next.js application backed by core AWS services. By the end, you'll have the confidence and experience to scaffold out your next great idea on AWS.
Looking for a fully managed GraphQL service?
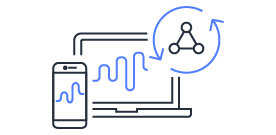
Explore AWS AppSync
AWS AppSync is an enterprise level, fully managed serverless GraphQL service with real-time data synchronization and offline programming features. AppSync makes it easy to build data driven mobile and web applications by securely handling all the application data management tasks such as real-time and offline data access, data synchronization, and data manipulation across multiple data sources.