Module 1: Introduction to AWS Lambda
LEARNING MODULE
Overview
In this tutorial you will learn how to use .NET with AWS Lambda. No previous knowledge of AWS Lambda is assumed, but you should have familiarity with AWS, and .NET. Over this course, you will learn to create, deploy, run, test, and debug .NET Lambda functions using the command line, Visual Studio, Visual Studio Code, and Rider.
In this introductory module, you will learn what serverless computing is, its benefits, and how this relates to AWS Lambda. This is followed by two examples of how easy it is to get started with .NET AWS Lambda functions.
Time to Complete
45 minutes
Serverless compute
Before talking about serverless computing, let's cover traditional computing for a moment.
Many companies, both small and large, use on premises servers, or data center based servers, to run their applications. When these companies need more servers, they have to buy them, physically install them, configure them, secure them, and maintain them. (In some cases, a data center may take care of physically installing them on your behalf, but you still need to do the rest.)
When all this is done, the company can deploy their applications to those servers. For many companies, this can take weeks or months. These delays have traditionally made it difficult to scale applications.
Cloud computing has made much of this easier. A company can launch an instance of a server (or multiple instances) in minutes. The company can create their own machine images with the virtual machine and operating system configured just the way they want. This reduces the amount of configuration that is needed once the server launches, but they still need to keep the server up to date with patches, security updates, and software updates, etc.
In many cases, these servers are often kept running 24/7, even though they are not in use all this time. The servers are often provisioned to handle the peak workload the application expects to see. But outside of this peak, the servers are at least partially idle. Appropriate scaling policies can be used to reduce help with this problem, but this can be hard to get right.
Also, the work of provisioning & maintaining the servers, and the time the servers spend idle, leads to costs that are not directly benefiting the company.
An alternative to this is serverless computing. There are no servers, there is no maintenance, and scaling is handled for you. You write your code, deploy it, and let AWS handle the infrastructure for you.
AWS offers two options for serverless computing, AWS Fargate for container based applications, and AWS Lambda for event driven applications.
AWS Lambda
AWS Lambda is a serverless compute service that executes your code in response to events received by the service. Events can be generated by other AWS services, such as SNS, SQS, S3, DynamoDB, API Gateway, etc.
Using an API Gateway, or Lambda function URLs allows you to trigger a Lambda function to run in response to an HTTP request. Lambda functions can also be invoked directly, though this too is considered an event.
You do not need to provision any infrastructure to use AWS Lambda. AWS also takes care of automatically scaling to meet the needs of your application, whether that is a few executions per day, or tens of thousands per second. The Lambda infrastructure, managed by AWS, is highly available, and fault tolerant.
With Lambda functions you only pay for the compute resources you use. When your code is not running, you are not paying.
You write your .NET applications using the IDEs you are already familiar with, then with the help of AWS tooling (or an IaC of your choice), you deploy your application to AWS Lambda.
When a Lambda function is invoked by an event, an execution environment is created, your initialization code is run (constructors, etc), then your code to handle the event is run.
Once execution is complete, the execution environment remains available for reuse for a non-deterministic period of time.
If another event arrives, the same execution environment is used, and the initialization code is not run again.
If no execution environment is available, a new one is created, and initialization code is run again.
AWS Lambda functions currently provide managed runtimes for .NET Core 3.1 and .NET 6. If you want to write applications in other versions of .NET, you can use Lambda custom runtimes, or a container based version of your application.
AWS provides C# and F# project templates for Lambda functions. These can be used from the command line, Visual Studio, Visual Studio Code, and Rider.
You can run your .NET Lambda function on x86_64 and arm64 processors. The choice of processor architecture has no impact on how you write your applications. Deploying to the processor of your choice is a simple matter of changing a single configuration parameter.
Main features of AWS Lambda
You don't need to learn a new language. Use your existing C# and F# knowledge to write your Lambda functions.
You can use Lambda functions with other AWS services, such as SNS, SQS, S3, Step Functions, and DynamoDB, to create event driven applications. Or perform custom tasks in response to events from these services.
If you need to orchestrate multiple running multiple Lambda functions, you can use AWS Step Functions. With Step Functions you create a state machine that manages the execution of your Lambda functions.
There is no infrastructure to provision or manage. AWS takes care of all of this for you. All you need to decide is the processor architecture, and the amount of memory to allocate to the function. Based on your choice of memory, AWS will choose the appropriate CPU speed, network bandwidth, and disk I/O.
Your Lambda functions are highly available, and fault tolerant, running in multiple availability zones in each region.
AWS Lambda functions start within milliseconds of an event arriving, and can scale quickly, and automatically, to match the volume of incoming events.
You only pay for what you use, and this is billed to the millisecond of use.
Lambda has a generous, always free tier, allowing you to run one million free requests per month and 400,000 GB-seconds of compute time per month
If you have bursty traffic patterns, Lambda will scale to meet those needs, and when the traffic reduces, you don't pay for the resources that are no longer needed.
Lambda is part of the Compute Savings Plans, which offers lower prices in return for a commitment use a certain amount of compute time over a one to three year period.
In the next section you will see how easy it is to get started with AWS Lambda. With just a few minutes work, you will have two .NET AWS Lambda functions running in the cloud
Two examples of how easy it is to get started with .NET AWS Lambda functions
Estimated time: 30 minutes
Please note, you may follow along with the examples presented here, but you do not need to.
If you have never worked with serverless technology, it may seem daunting at first. Even knowing how to get started can be hard to figure out.
By the end of this short module, you will have two .NET Lambda functions up and running on AWS.
One you invoke from the command line, the other via a public HTTPS endpoint that you will setup with almost no effort.
You will use the command line to create, build and deploy both examples.
Prerequisites
1. An AWS account.
If you do not have an AWS account, please see this page on creating and activating an account. When you create a new account, a free tier is automatically activated for you. The free tier provides free usage of certain AWS services up to a limit every. Learn more about the free tier here.
2. An installed and configured AWS CLI.
If you don’t have the AWS CLI, please download and install it. Then configure the AWS CLI with your credentials and default region. Your choice of default region will depend on your geographic location and the AWS services you want to use. Service availability varies by region.
The AWS user should have the AdministratorAccess policy attached, see the section - A note on permissions, in module 3 for more details.
3. The .NET 6 SDK
If you don’t have .NET 6 SDK installed, download and install it.
Get the tools and templates
A later module will explain more about the available tooling and templates, but for now you can get started by running the two commands below:
dotnet tool install -g Amazon.Lambda.Tools
dotnet new -i Amazon.Lambda.Templates
Demo 1: The "Hello World!" of Lambda functions
This is the most basic Lambda function you can write that takes some input and returns a response, and you will have it up and running in a few minutes.
Step 1 - Create a .NET project
From the command line/terminal window, run the following command to create a new Lambda function.
dotnet new lambda.EmptyFunction -n HelloLambda
Step 2 - Deploy the Lambda function
Change to the HelloLambda/src/HelloLambda directory:
cd HelloLambda/src/HelloLambda
If you are interested, open up the project in this directory. You will see a file named Function.cs. That is where the code that executes your function is located. Don’t change anything in the file for now.
From the command line, run the following command to deploy your function:
dotnet lambda deploy-function --function-name HelloLambda
You will see output that looks like the below.
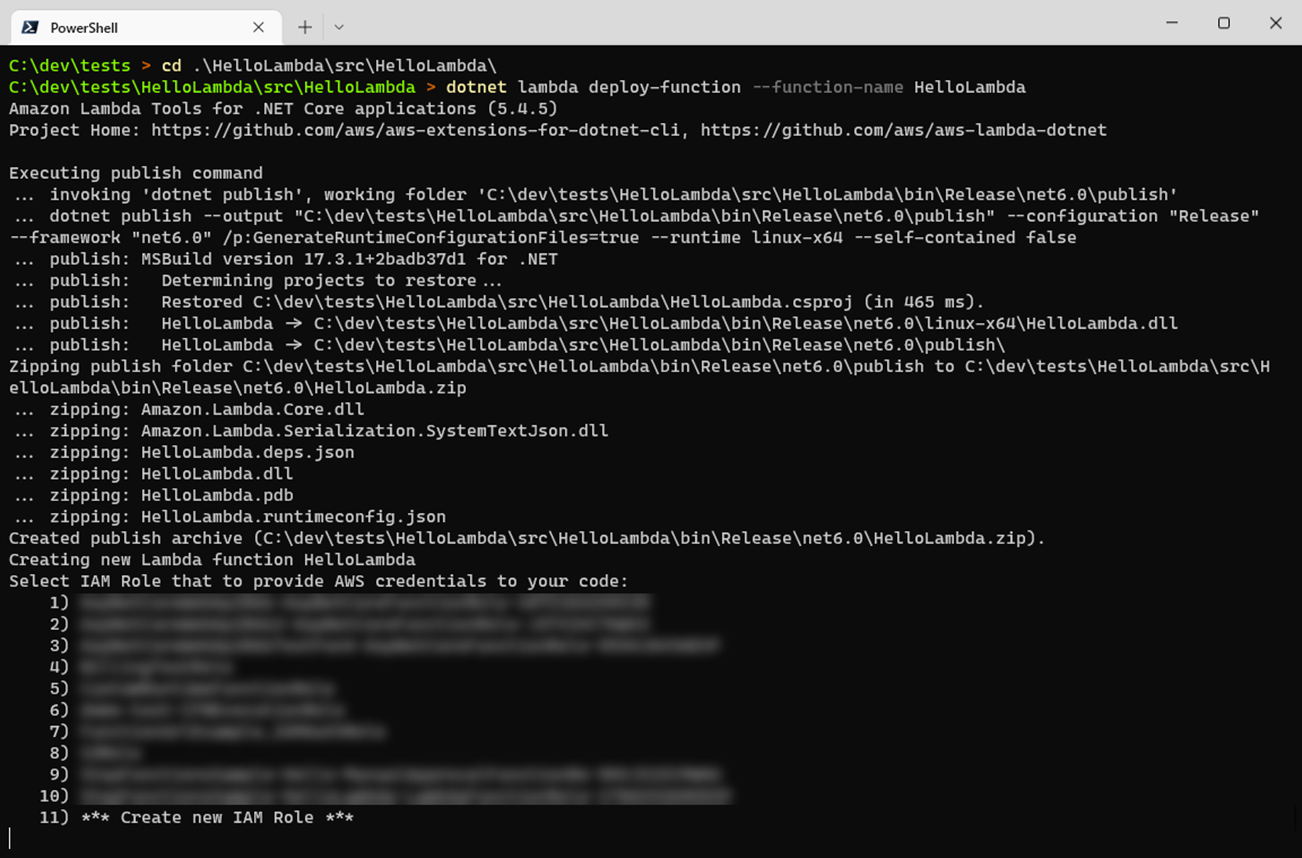
The AWS Toolkit for Visual Studio does not support working with your ECR public registry, or enabling features such as automated scanning and repository encryption for new repositories in your private registry. If those features are required, create repositories using the AWS Management Console or command-line tools such as the AWS CLI and AWS Tools for PowerShell.
At this point, one of two things will happen.
- If there are no roles in your AWS account, you will be asked to "Enter name of the new IAM Role", do so. Use the name HelloLambdaRole.
- If there are roles in your AWS account, you will be shown a list, but at the bottom will Create new IAM Role, type in the number associated with that. Then you will be asked to "Enter name of the new IAM Role", do so. Use the name HelloLambdaRole.
Next, you will see a list of permissions that can be attached to the role you are creating. Choose AWSLambdaBasicExecutionRole. It is number 6 on my list.
Wait a few seconds as the role, permissions, and Lambda function are created.
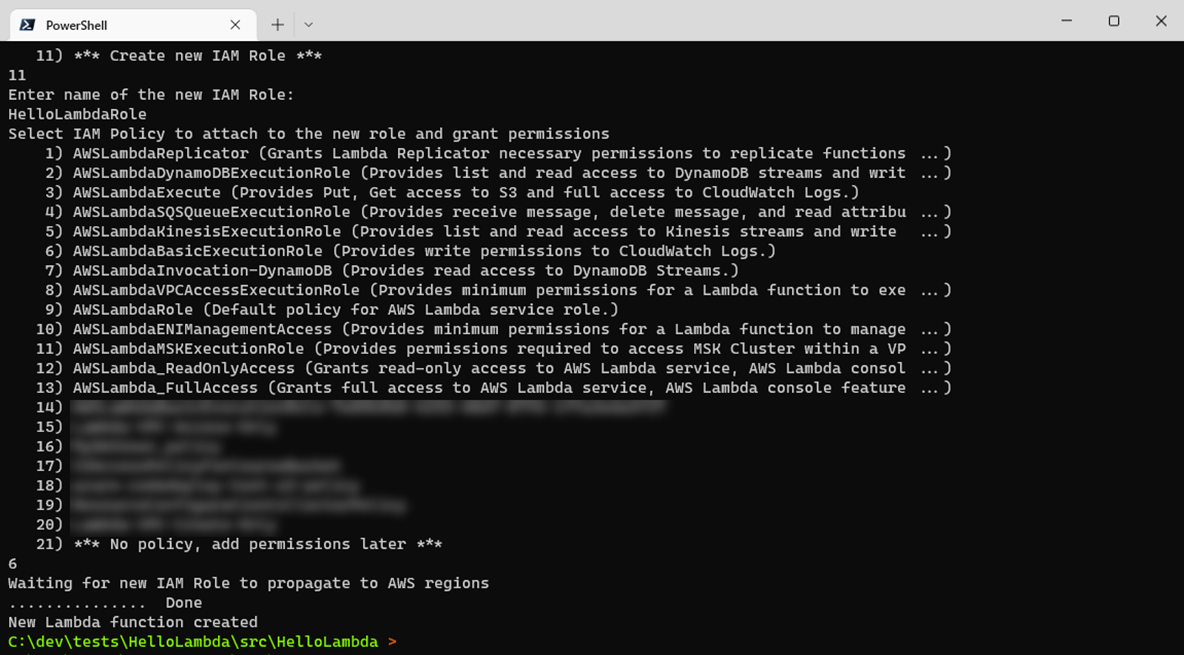
Step 3 - Invoke the Lambda function
Step 4 - Clean up
With Lambda functions, you are not charged when they are not running, however with other AWS services you will be changed even when the service is idle. It is always good practice to remove resources you are not using.
Run the following command to delete the Lambda function:
dotnet lambda delete-function --function-name HelloLambda
Note, this does not delete the role you created, only the function.
Demo 2: A .NET API running in a Lambda function
In this demo you will create a .NET API application that handles HTTPS requests. You can use the newer minimal API template, or the older full API with controllers template - the steps will be the same. If you are familiar with .NET 6 top level statements and API endpoints, consider using the minimal template - serverless.AspNetCoreMinimalAPI. If you are not familiar with top level statements, use the older template - serverless.AspNetCoreWebAPI.
Step 1 - Create a .NET project
If you want to use the minimal API template, run the following command:
dotnet new serverless.AspNetCoreMinimalAPI -n HelloLambdaAPI
If you are more familiar with the full API template with controllers, run the following command:
dotnet new serverless.AspNetCoreWebAPI -n HelloLambdaAPI
Step 2 - Create an S3 bucket
This is a one-off step. The command in step 3 needs an S3 bucket to store files related to the deployment.
You need to create a bucket name that is unique, across all AWS accounts (not just yours), and across all regions.
If you are using us-east-1, run the following command:
aws s3api create-bucket --bucket your-unique-bucket-name1234
If you want to use a different region, run:
aws s3api create-bucket --bucket your-unique-bucket-name1234 --create-bucket-configuration LocationConstraint=REGION
If the bucket name you select is in use, you'll receive the following error:
An error occurred (BucketAlreadyExists) when calling the CreateBucket operation: The requested bucket name is not available. The bucket namespace is shared by all users of the system. Please select a different name and try again.
Step 3 - Deploy the Lambda function
Change to the HelloLambdaAPI/src/HelloLambdaAPI directory.
From the command line, run the following command to deploy your function:
dotnet lambda deploy-serverless --stack-name HelloLambdaAPI --s3-bucket your-unique-bucket-name1234
Sit back and wait...
You will see some output related to the build and deployment process, and at the end there will be a link:
Output Name Value
------------------------------ --------------------------------------------------
ApiURL https://xxxxxxxxxx.execute-api.us-east-1.amazonaws.com/Prod/
Open that in a browser. Depending on your choice of project template, you will see one of the messages below:
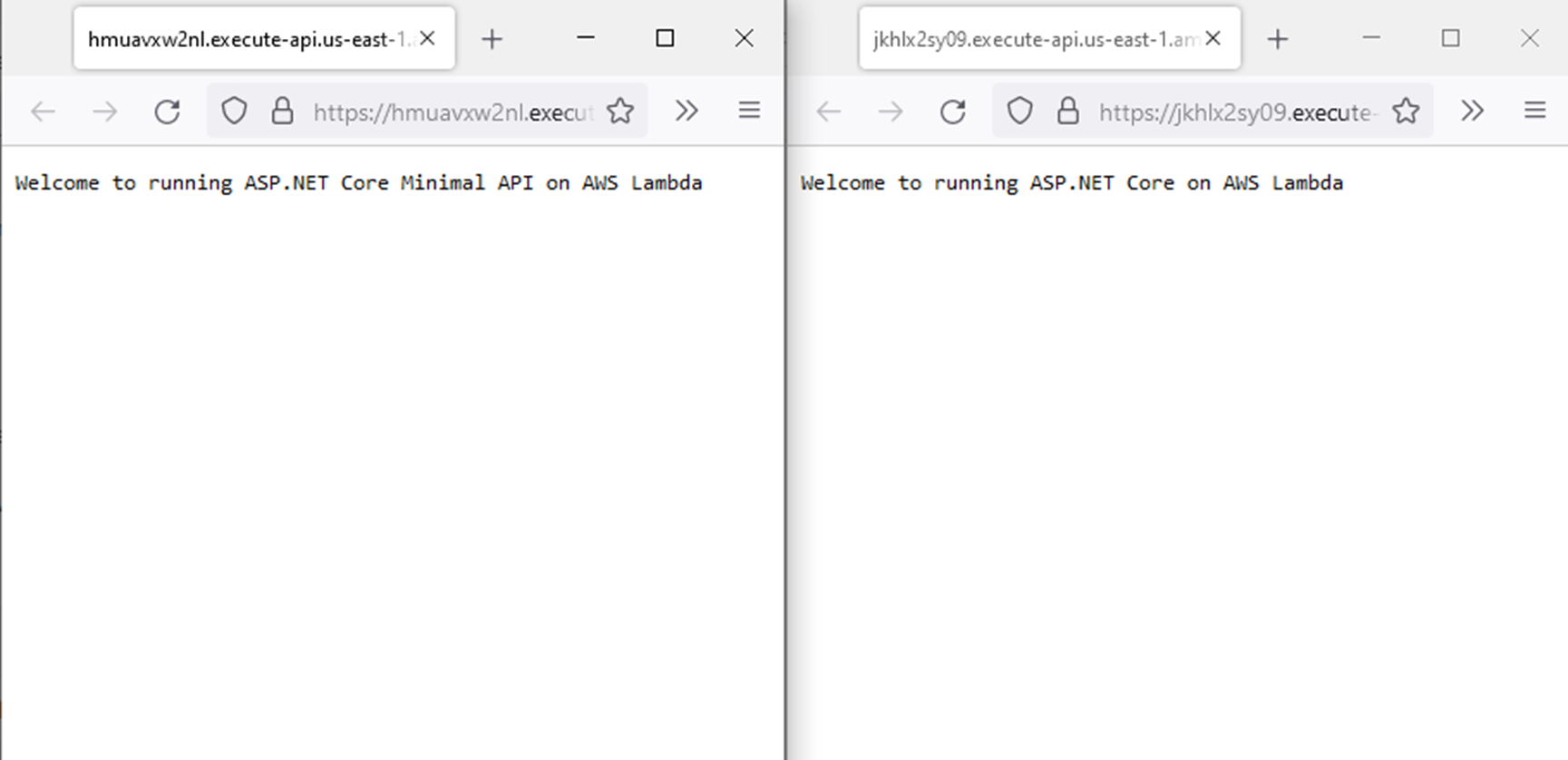
Step 4 - Clean up
To remove the Lambda function and associated resources, run the following command:
dotnet lambda delete-serverless HelloLambdaAPI
Conclusion
There you go. In just a few minutes, you have deployed two Lambda functions. That’s how easy it is to get started with .NET Lambda functions!