AWS News Blog
Sweet Treats for DynamoDB Users
My friends on the DynamoDB team sent me a tasty bribe (courtesy of Seattle’s Trophy Cupcakes) in a successful attempt to get me to tell you about some features that I didn’t have time to tell you about earlier this month!
As you may know from my previous post, we launched support for JSON, increased the maximum size of a DynamoDB item, and expanded the AWS Free Tier to 25 GB of storage and up to 200 million requests.
Here’s what I left out, and what I’d like to tell you about today:
- JavaScript Web Shell – You can use DynamoDB Local, the new Web Shell, and a hands-on tutorial to get started quickly.
- SQL-Like Expressions – This taste of SQL within a NoSQL database makes the APIs more familiar and more expressive while also improving the readability of the code.
- Improved Conditions – Our new and more expressive server-side filtering reduces the amount of data transferred to the client and also reduces overhead for conditional updates.
JavaScript Web Shell
DynamoDB Local now comes with a free and very helpful web-based user interface known as the DynamoDB JavaScript Shell. You simply download the DynamoDB Local archive file and extract the contents to the directory of your choice. Then you launch DynamoDB Local like this:
C:\temp\L> java -Djava.library.path=./DynamoDBLocal_lib -jar DynamoDBLocal.jar
The Web Shell is accessible as /shell on port 8000 (you can specify another port on the command line if necessary):
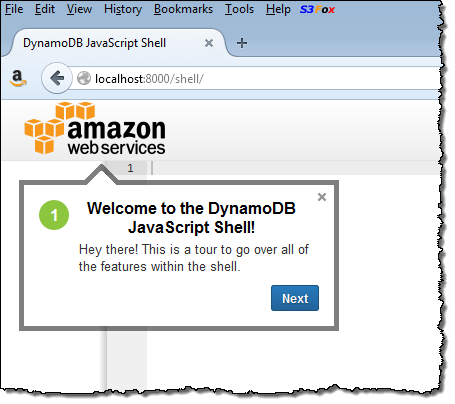
The Web Shell looks like this (code editor on the left, console output on the right):
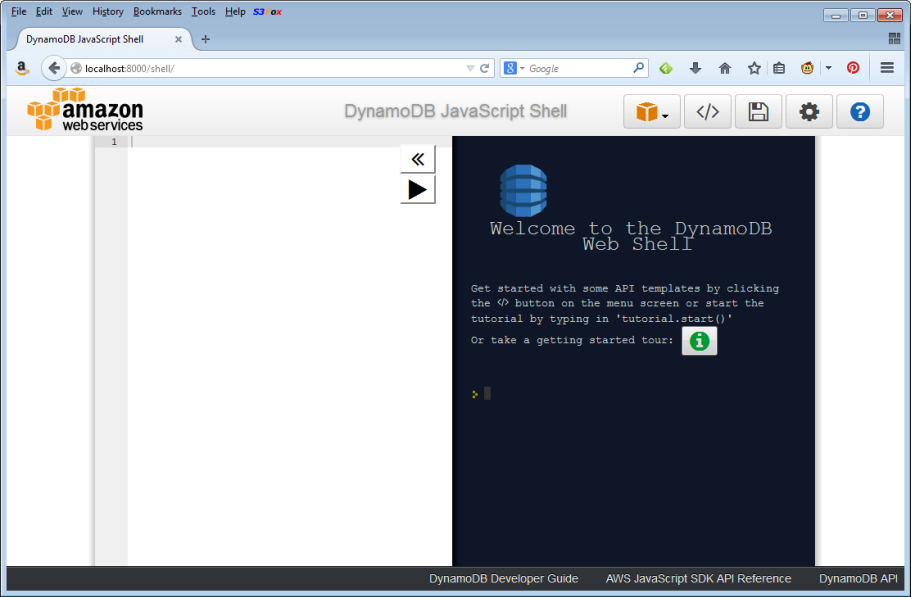
I simply enter my JavaScript on the list and click on the Run button to execute it. Here’s how I would create a table:
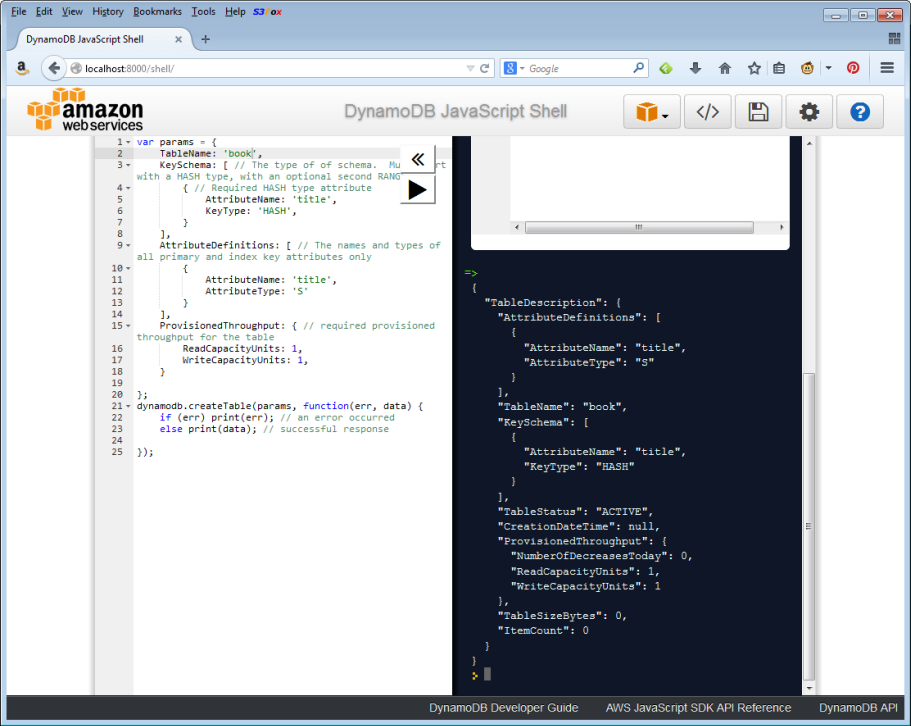
The shell contains a template for each method in the DynamoDB API. I can click the “Control and Space
keys simultaneously to display possible completions of the current command.
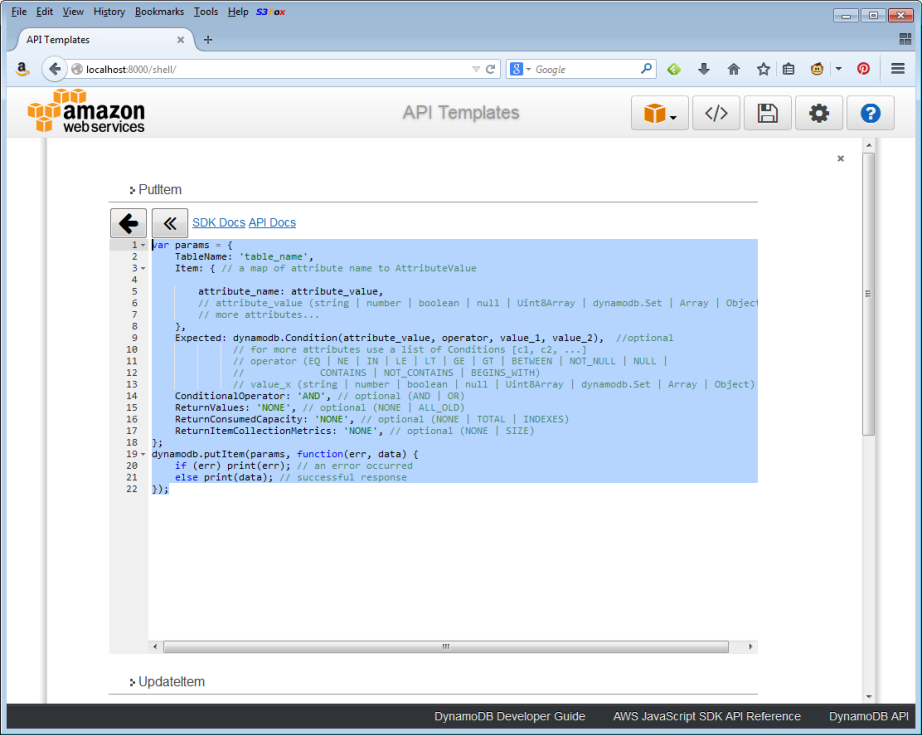
It is easy to create a table using the AWS SDK for JavaScript in the Browser:
var params = {
TableName: 'book',
KeySchema: [
{
AttributeName: 'title',
KeyType: 'HASH',
}
],
AttributeDefinitions: [
{
AttributeName: 'title',
AttributeType: 'S'
}
],
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1,
}
};
dynamodb.createTable(params, function(err, data) {
if (err) print(err); // an error occurred
else print(data); // successful response
});
Inserting new items in to the table is a breeze:
var params = {
TableName: 'book',
Item: { // a map of attribute name to AttributeValue
title: "Sample Application: CloudList",
chapter: 10
}
};
dynamodb.putItem(params, function(err, data) {
if (err) print(err); // an error occurred
else print(data); // successful response
});
Scanning the table is also very easy. Here’s how to retrieve the first page of results:
var params = {
TableName: 'book',
};
dynamodb.scan(params, function(err, data) {
if (err) print(err); // an error occurred
else print(data); // successful response
});
Here’s what that looks like in the Web Shell:
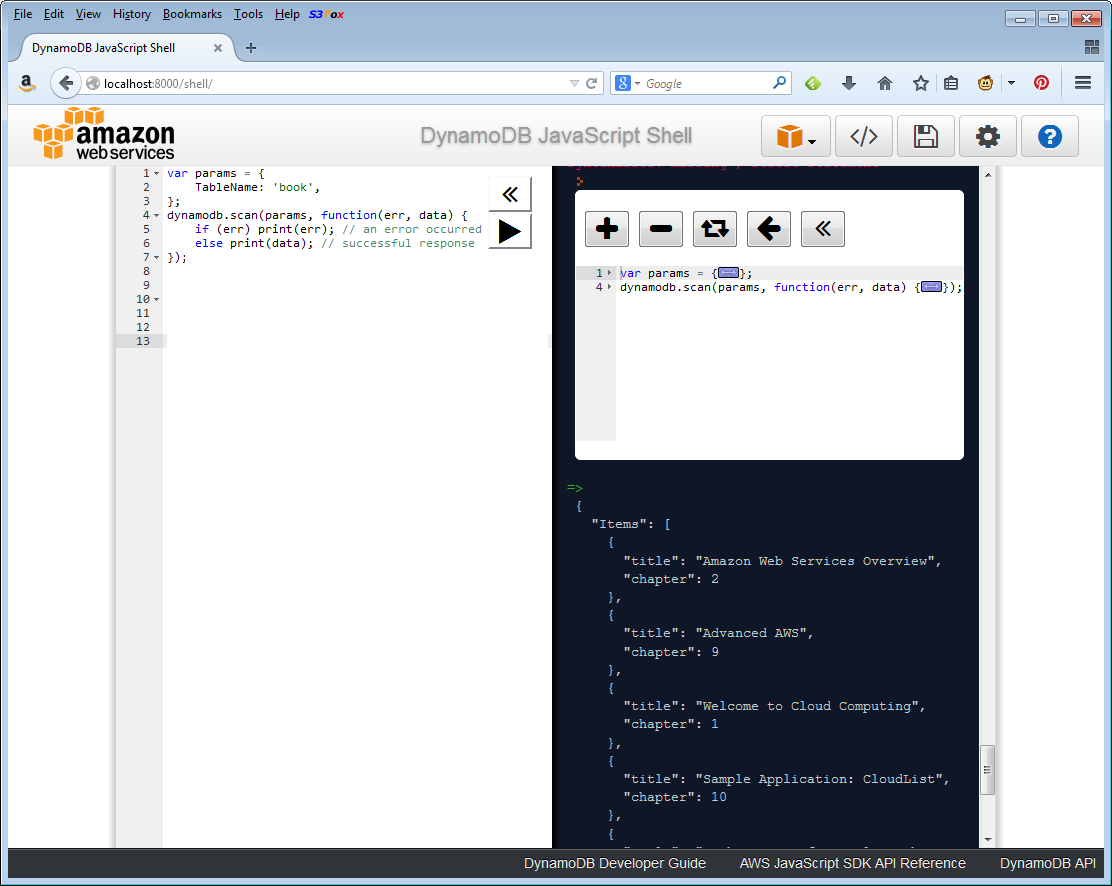
The DynamoDB Web Shell is available to you at no charge as part of DynamoDB Local!
SQL-Like Expressions
DynamoDB’s Scan
and Query
methods now accept a simple and familiar query language reminiscent of SQL. Here is how you would scan a table to find all URLs that start with “long.example.com/” and were tagged on or after “2014-09”:
dynamodb.scan({
TableName: 'Url',
ProjectionExpression: 'ShortUrl, RedirectTo, Metadata.CreatedBy',
FilterExpression:
'begins_with(RedirectTo, :domain) ' +
'AND Metadata.CreateDate >= :start_date',
ExpressionAttributeValues: {
':domain': 'long.example.com/',
':start_date': '2014-09'
}
}).eachPage(function(err, data) {
if (err) print(err);
else if (data) print(data);
});
Improved Conditions
Earlier this year, we launched support for inequalities in scan filter, query filter, and conditional writes. Now, you get the full expressiveness of SQL-like WHERE clauses, with nested parenthesis, AND, OR, and NOT. You can now specify the same attribute multiple times, and you can even compare existing attribute values to each other (none of which was possible with the previous API).
For example, this JavaScript snippet scans a “Short URL” table. looking for all URLs that have reached their target for page views and that either include the “map” tag or resolve to “long.example.com”:
dynamodb.scan({
TableName: 'Url',
FilterExpression:
"(contains(Tags, :tag)) " +
"OR begins_with(RedirectTo, :domain)) " +
" AND " +
"Stats.PageViews > Metadata.TargetViews",
ExpressionAttributeValues: {
":tag": "map",
":domain": "long.example.com"
}
}).eachPage(function(err, data) {
if (err) print(err);
else if (data) print(data);
});
— Jeff;