Front-End Web & Mobile
Add Amazon Bedrock Chat Capabilities to a React Native App
This post will teach you to create a React Native chat application using Amazon Bedrock to build AI-powered chat features.
The sample application includes the following:
- A mobile application using custom JSX components for the user interface.
- Seamless end-user chat interactions integrated with Amazon Bedrock foundational models.
- Backend development powered by AWS Amplify Gen2.
- Implementation of RESTful APIs using Amazon API Gateway for communication.
- Serverless backend processing using AWS Lambda.
- Use Amazon CloudWatch Logs to monitor AWS Lambda functions and view their logs.
Architecture
Figure 1 illustrates the architecture and process flow for running a React Native mobile application backend in the AWS Cloud.
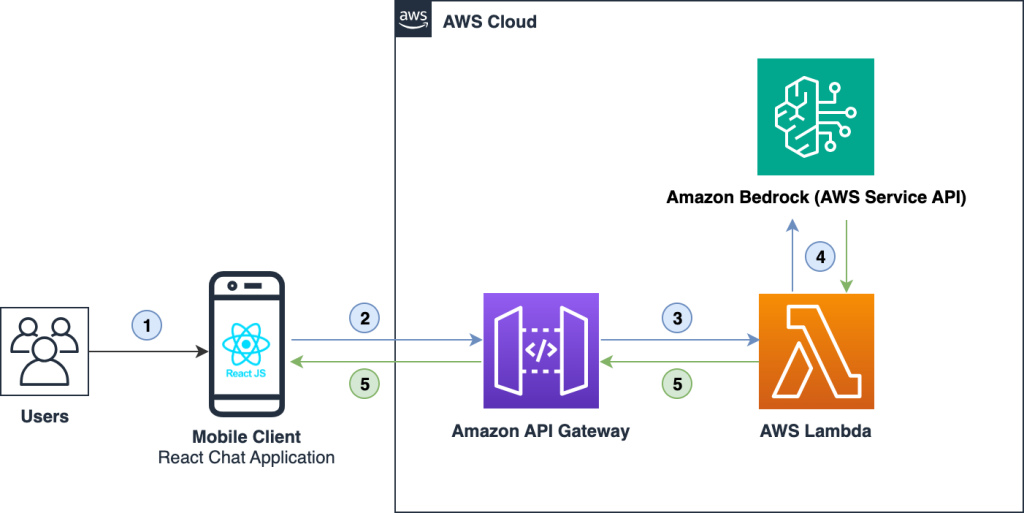
Figure 1: Flow of a mobile chat application using AWS services.
- Users access the application from their mobile devices.
- The mobile client React chat application interfaces with Amazon API Gateway.
- Amazon API Gateway interacts with AWS Lambda to post and fetch the data.
- AWS Lambda interacts with one of the Amazon Bedrock models and returns the generated response in a JSON format.
- The processed data is then returned to the frontend for display, facilitating content or chat presentation.
Note: This tutorial was built on a Macintosh and uses the macOS terminal for command execution. If you are using either Windows or Linux operating system, use the equivalent commands.
Prerequisites
Before you get started, make sure you have the following installed:
- Create an AWS Account if you don’t have one. Note that AWS Amplify is part of the AWS Free Tier.
- Configure your AWS account to use with Amplify.
- Node.js v18.17 or later
- npm v9 or later
- git v2.14.1 or later
- Expo CLI
- Expo Go is a sandbox that enables you to quickly experiment with building native Android and iOS apps. It’s the fastest way to get started.
Create your application
Run the below command to create an app (Uses Expo‘s TypeScript template).
Start your application
Navigate to the root folder of your application and execute the following command to start the application.
When prompted, enter i
to open the App in iOS simulator.
Upon successful execution, you will see your new application running in the iOS Simulator, as shown in Figure 2.
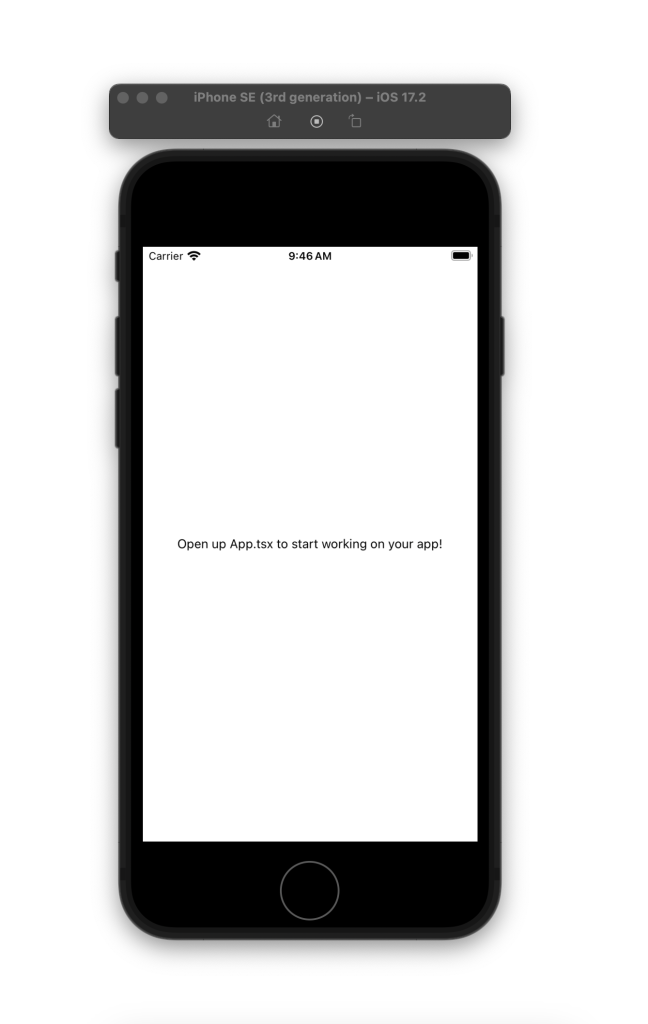
Figure 2: New application running in the iOS Simulator
Set up the backend environment
Open a new terminal window from the root project directory. Initialize Amplify by executing the following command.
Note: TypeScript is not a requirement but is recommended for an optimal experience.
Install packages/libraries
Keep the code snippets handy
The complete code snippets are available at amazon-bedrock-react-native-chat-app, keep them ready for easy copying and pasting.
Open the project root with the IDE of your choice.
Create backend entry point for your backend
To create the backend.ts file and include the provided code, follow these steps.
- Inside the amplify folder, create a new file named backend.ts.
- Copy the entire code snippet from the demo repository for the backend.ts component and paste it into your newly created backend.ts file.
This code implements the following.
- Defines a backend environment with a Lambda function.
- Creates an API stack and a REST API with logging and CORS settings.
- Integrates the Lambda function with a POST method at the /chat endpoint.
- Sets up an IAM policy to allow API invocation and access to a Bedrock model.
- Adds API endpoint details to the backend output for configuration.
Note: For this tutorial, the API is open to unauthenticated access. Consider implementing authentication and authorization if needed.
Set up Amplify REST API with Lambda Function
Create a new directory and a resource file amplify/functions/api-function/resource.ts. Then, define the function with defineFunction:
Create the corresponding handler file, amplify/functions/api-function/handler.ts. Copy the entire code snippet from the demo repository for the handler.ts file and paste it into your newly created handler.ts file.
This code implements the following.
- Imports the necessary libraries.
- Configures the Amazon Bedrock Runtime.
- Utilizes the AI21 model for this demo application.
- Extracts the message input from body if provided, else uses the default message.
- Invokes the Amazon Bedrock model with set of parameters.
- Processes and returns the response.
Now you can run npx ampx sandbox
to create your first backend!
Amplify Gen 2 requires your backend to be configured for use with ECMAScript modules (ESM). Create a local file in the Amplify backend directory, amplify/package.json
Frontend development
Make the following changes in the frontend code.
Create header component
To create the Header.jsx file and include the provided code, follow these steps.
- Inside the src/components/ folder, create a new file named Header.jsx.
- Copy the entire code snippet from the demo repository for the Header.jsx component and paste it into your newly created Header.jsx file.
Now, you have successfully created the Header.jsx component that displays a header with a customizable title.
Create avatar component
- Inside the src/components/ folder, create a new file named Avatar.jsx.
- Copy the entire code snippet from the demo repository for the Avatar.jsx component and paste it into your newly created Avatar.jsx file.
The Avatar component dynamically renders avatars for the user and chat bot.
Create ChatInput component
- Inside the src/components/ folder, create a new file named ChatInput.jsx.
- Copy the entire code snippet from the demo repository for the ChatInput.jsx component and paste it into your newly created ChatInput.jsx file.
The ChatInput component provides a user interface for entering and sending messages in a chat application. It consists of a text input field for typing messages, accompanied by a Send button to trigger the message sending action.
Create message bubble component
- Inside the src/components/ folder, create a new file named MessageBubble.jsx.
- Copy the entire code snippet from the demo repository for the MessageBubble.jsx component and paste it into your newly created MessageBubble.jsx file.
The MessageBubble component renders chat message bubbles in a chat application, dynamically styling them based on the sender (user or bot). It integrates user and chat bot avatars alongside the message text, offering a visually distinct and organized representation of chat interactions.
Create chat component
- Inside the src/components/ folder, create a new file named Chat.jsx.
- Copy the entire code snippet from the demo repository for the Chat.jsx component and paste it into your newly created Chat.jsx file.
The chat component functionality:
- Manages chat messages and user input.
- Uses state to store messages and the current input.
- Handles sending messages, fetches bot responses from an API, and renders messages using MessageBubble.
- The user interacts through the integrated ChatInput.
Create API service
- Inside the src/services/ folder, create a new file named api.js.
- Copy the entire code snippet from the demo repository for the api.js component and paste it into your newly created api.js file.
- Replace the apiUrl with Amazon API Gateway provided URL.
The above code defines an async function, fetchChatAPI, that sends a POST request to a REST API endpoint using AWS Amplify. It processes the response to extract and clean a specific text field, handling errors by logging the details.
Update the App component
To update the App.tsx component and incorporate the provided code, follow these steps.
- Navigate to the src folder and locate the App.tsx file.
- Copy the entire code snippet from the demo repository for the App.tsx component and paste it into your existing App.tsx file.
Run your React Native application in the simulator, if is not running already.
The App.tsx component is updated with the provided code. You can open the app in the simulator, enter text, select the Send button, and receive responses from the Amazon Bedrock model. Make sure to check the provided screenshot (Figure 3) as a reference for the expected output.
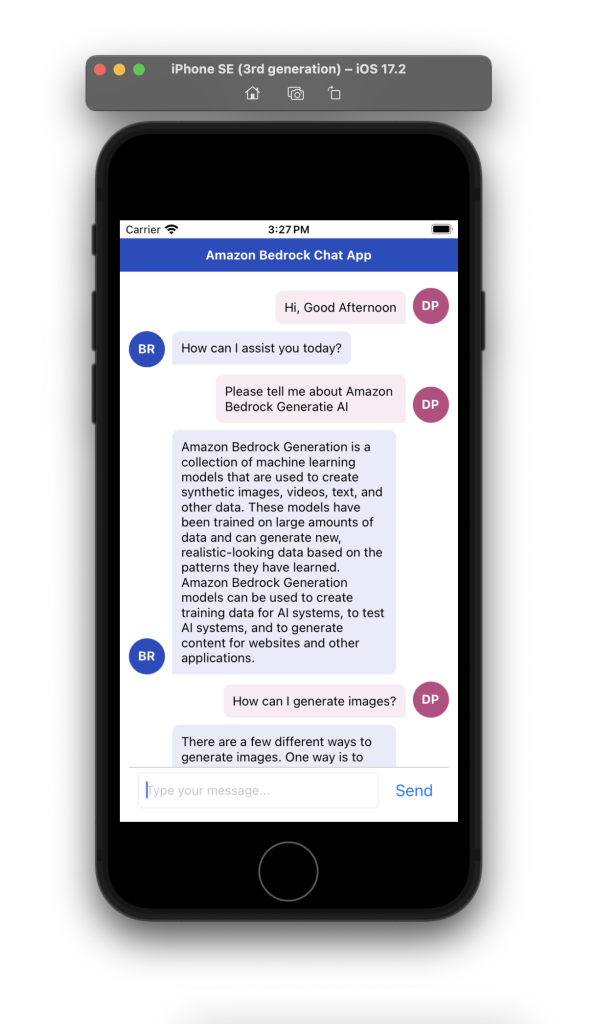
Amazon Bedrock chat application user interface
Conclusion
In this blog post, we have guided you through the development of a simple chat application for the mobile platform using Amazon Bedrock, which leverages generative AI models. By integrating We’ve also used multiple AWS services including AWS Amplify, Amazon API Gateway, and AWS Lambda. To learn more, go to the AWS Amplify Documentation.