AWS Contact Center
Making Cold Transfers Using the Amazon Connect Streams API
In most contact centers, the agents’ time is the most valuable commodity. Contact center supervisors
make the most of that time by reducing the average handle time of interactions, so that agents can
quickly address customer issues and move on to the next interaction.
This approach has a broader impact on contact center key performance indicators such as the average
speed of answer, average hold times, abandoned rates, etc. Supervisors must maintain a fine balance of
ensuring high customer satisfaction and reducing the time it takes to address customer issues. At times,
agents must consult other parties to solve customer issues. Those other parties could involve external
vendors, internal colleagues, or supervisors.
Amazon Connect natively supports warm transfers, which involve putting the customer on hold as the
agent makes an introduction about the caller to another party. Then, the agent can conference all
parties together and pass the context of the call for a seamless customer experience. The agent can then
leave this interaction, and the other parties stay connected. This approach works great for high-touch
customer experiences.
However, some calls don’t need their context passed on to the receiving party. Agents and supervisors
want to quickly transfer these calls and move on to the next interaction. This is also known as a cold
transfer. In this post, I demonstrate cold transfers using the Amazon Connect Streams API. For more
information about customizing the contact control panel (CCP), see the Streams API GitHub repository.
This post discusses the following cold transfer scenarios. In the first three scenarios, the agent is
connecting multiple external parties. In the fourth and fifth scenarios, the agent is connecting an
external party to another queue or agent.
- The agent makes an outbound call and then makes another outbound call. The agent cold
transfers the first call to the second call. - The agent receives an incoming call and then makes an outbound call. The agent cold transfers
the first inbound call to the second outbound call. - The agent receives an incoming call, then cold transfers that call to the external number using
quick connect. - The agent receives an incoming call or makes an outbound call, then cold transfers that call to
another queue using quick connects. - The agent receives an incoming call or makes an outbound call, then cold transfers that call to
another agent using quick connects.
Background
When an agent makes or receives a phone call, Amazon Connect creates a contact object. That contact
object initially possesses two connections:
- The agent connection to the Connect instance
- The calling or called party.
When the agent makes another phone call to an external number or quick connects, Amazon Connect creates a new connection and adds it to the same contact object. Except for cold transfers to agent quick connects, the agent connection can then safely disconnect and the remaining two parties remain conferenced automatically. For a cold transfer to agent quick connects, the destination agent must accept the phone call. Otherwise, the call fails. In this post, I review a workaround.
Solution
In a production environment, you can implement these API calls in a custom CCP. However, if you are looking for quick validation, you can also make these API calls directly from the console in the Developer Tools section of Amazon Connect supported browsers.
Prerequisites
Before getting started with this walk through, you need the following resources in place:
- An AWS account
- Amazon Connect Instance
- A configured Amazon Connect contact center and agents
- (Optional) Quick Connects for external, agents and queue transfers
- (Optional) A Custom CCP
- Whitelisted Integrated Application
Connecting multiple external parties (scenarios 1–3)
For scenarios 1 through 3—where both parties are external, the solution is similar. The connection type differs if it is an inbound or outbound call, but connection type has no bearing on implementing cold transfers.
Use the Streams API to make the first outbound call:
var agent = new lily.Agent();
agent.connect(connect.Endpoint.byPhoneNumber("+1xxxxxxxxxx"),{});
Replace the phone number “+1xxxxxxxxxx” with an actual destination phone number.
You can use an agent snapshot to confirm that both connections for that contact successfully connected.
agent.toSnapshot();
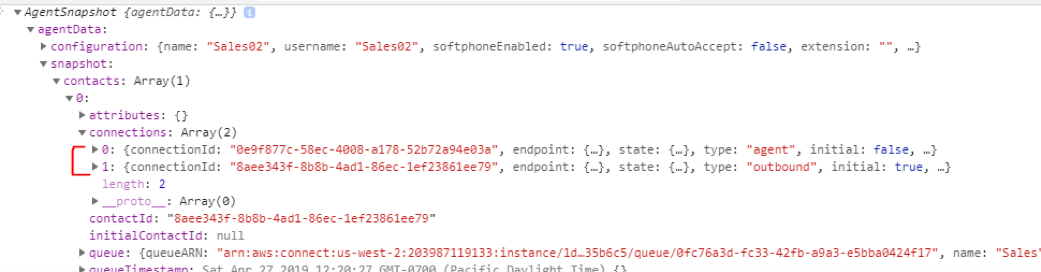
Agent Snapshot
Create another endpoint of the type phone number. Add a connection to that endpoint. This puts the first call on hold and initiates a connection to a second destination. Replace the phone number “+1xxxxxxxxxx” with an actual destination phone number.
var endpoint = connect.Endpoint.byPhoneNumber("+1xxxxxxxxxx");
agent.getContacts(lily.ContactType.VOICE)[0].addConnection(endpoint, {
success: function(data) {
alert("transfer success");
},
failure: function(data) {
alert("transfer failed");
}
});
You can use the agent snapshot again to verify that the third connection is connected.
agent.toSnapshot();
After that, obtain the agent connection of this contact object and destroy it.
You can copy the contact object from the agent snapshot, or obtain it explicitly using the following method:
var contact = agent.getContacts(lily.ContactType.VOICE)[0]
contact.getAgentConnection().destroy();
This completes the cold transfer to external numbers, either through quick connect for external transfer or agent manually dialed the number.
Connecting to another queue (scenario 4)
In Scenario 4, the agent makes an outbound call or receives an incoming call, and then cold transfers it to another queue using queue quick connect.
Use the Streams API to make the first outbound call:
var agent = new lily.Agent();
agent.connect(connect.Endpoint.byPhoneNumber("+1xxxxxxxxxx"),{});
At this time, you can use an agent snapshot to confirm that both connections for that contact successfully connected.
agent.toSnapshot();
You can quickly view all the quick connect endpoints for this agent:
agent.getEndpoints(agent.getAllQueueARNs(),{success: function(data){console.log(data.endpoints);},failure:function(){}});
For this example, assume that the quick connect index for desired queue is 9:
Var quick;
agent.getEndpoints(agent.getAllQueueARNs(),{success: function(data){quick=data.endpoints[9];},failure:function(){}});
agent.getContacts(lily.ContactType.VOICE)[0].addConnection(quick, {
success: function(data) {
alert("transfer success");
},
failure: function(data) {
alert("transfer failed");
}
});
This places the first call on hold and adds a third connection to this contact. The first connection is the agent, and the second connection is the customer on hold. Again, confirm the state of your call using an agent snapshot.
agent.toSnapshot();
At this point, destroying the agent connection puts the other parties in a conference call. The customer experiences whatever you configure in the queue transfer contact flow. If their call is waiting in the queue, they experience the customer hold flow.
var contact = agent.getContacts(lily.ContactType.VOICE)[0]
contact.getAgentConnection().destroy();
Connecting to another agent (scenario 5)
Scenario 5 is similar to scenario 4. It uses the same quick connect endpoints and adds a connection to an agent quick connect.
However, if the destination agent is not available and you must make a cold transfer, the customer is disconnected, thanks to the transfer to agent block in the contact flow.
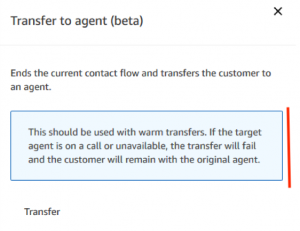
Amazon Connect Transfer to Agent Contact Flow Block
To deal with this situation, coach your agents as follows: For cold transfers to other agents, they must wait for the destination agent to connect before completing the cold transfer.
If that is not possible, one way to solve for this scenario could be by adopting the following workaround:
- Create a Queue transfer contact flow and set the working queue of the desired agent destination.
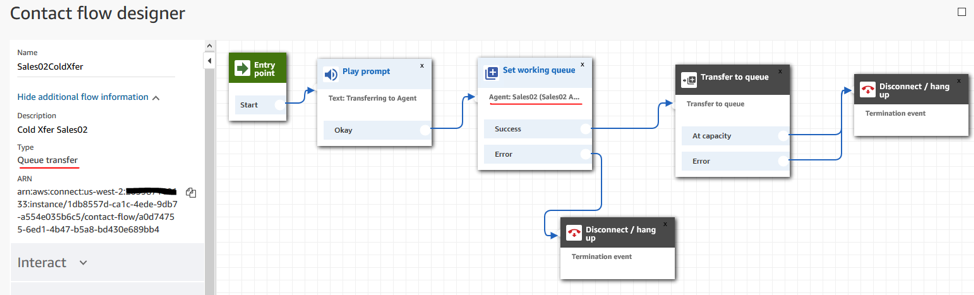
Queue Transfer Contact low
- Create a Queue quick connect called ColdXferSales02. Assign the contact flow to this quick connect.
- Add the ColdXferSales02 quick connect to the queue tied to the agent using the routing profile.
With this done, you can use the same solution described in scenario 4 for this cold transfer to an agent. If the agent is unavailable, the customer waits in the queue until that agent becomes available. Make certain that you have the following procedure in place in case an agent is unavailable:
var agent = new lily.Agent();
agent.connect(connect.Endpoint.byPhoneNumber("+1xxxxxxxxxx"),{});
Use an agent snapshot to confirm that both connections for that contact successfully connected:
agent.toSnapshot();
You can view all quick connect endpoints for this agent:
agent.getEndpoints(agent.getAllQueueARNs(),{success: function(data){console.log(data.endpoints);},failure:function(){}});
For this example, assume that the quick connect index for the desired queue is 9:
Var quick;
agent.getEndpoints(agent.getAllQueueARNs(),{success: function(data){quick=data.endpoints[9];},failure:function(){}});
agent.getContacts(lily.ContactType.VOICE)[0].addConnection(quick, {
success: function(data) {
alert("transfer success");
},
failure: function(data) {
alert("transfer failed");
}
});
This places the first call on hold and adds a third connection to this contact. Confirm that the third connection connected with an agent snapshot:
agent.toSnapshot();
Destroying the agent connection at this point puts the other parties in a conference call. The customer experiences whatever you configure in the queue transfer contact flow or the customer hold flow if their call is waiting in the queue.
var contact = agent.getContacts(lily.ContactType.VOICE)[0]
contact.getAgentConnection().destroy();
Conclusion
In this post, you learned how to implement cold transfers using the Amazon Connect Streams API. The Streams API methods described in this post enable your agents to transfer calls quickly to other destinations and move on to servicing their next customer.
You can use the built-in warm transfer capabilities of Amazon Connect for high-touch customers and intricate issues that require passing along caller context and making introductions. In other cases, your agents can quickly transfer the calls and move on to the next interactions in the queue.
Test these scenarios with the developer tools of your preferred supported browser. Paste the code in this post in the browser console for quick validation, or make it part of your production implementation. To learn more, see Getting Started with Amazon Connect. To learn about other exposed event subscription methods and action methods exposed, see the Amazon Connect Streams API documentation.