AWS Compute Blog
Building Loosely Coupled, Scalable, C# Applications with Amazon SQS and Amazon SNS
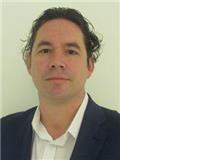
Stephen Liedig, Solutions Architect
One of the many challenges professional software architects and developers face is how to make cloud-native applications scalable, fault-tolerant, and highly available.
Fundamental to your project success is understanding the importance of making systems highly cohesive and loosely coupled. That means considering the multi-dimensional facets of system coupling to support the distributed nature of the applications that you are building for the cloud.
By that, I mean addressing not only the application-level coupling (managing incoming and outgoing dependencies), but also considering the impacts of of platform, spatial, and temporal coupling of your systems. Platform coupling relates to the interoperability (or lack thereof) of heterogeneous systems components. Spatial coupling deals with managing components at a network topology level or protocol level. Temporal, or runtime coupling, refers to the ability of a component within your system to do any kind of meaningful work while it is performing a synchronous, blocking operation.
The AWS messaging services, Amazon SQS and Amazon SNS, help you deal with these forms of coupling by providing mechanisms for:
- Reliable, durable, and fault-tolerant delivery of messages between application components
- Logical decomposition of systems and increased autonomy of components
- Creating unidirectional, non-blocking operations, temporarily decoupling system components at runtime
- Decreasing the dependencies that components have on each other through standard communication and network channels
Following on the recent topic, Building Scalable Applications and Microservices: Adding Messaging to Your Toolbox, in this post, I look at some of the ways you can introduce SQS and SNS into your architectures to decouple your components, and show how you can implement them using C#.
Walkthrough
To illustrate some of these concepts, consider a web application that processes customer orders. As good architects and developers, you have followed best practices and made your application scalable and highly available. Your solution included implementing load balancing, dynamic scaling across multiple Availability Zones, and persisting orders in a Multi-AZ Amazon RDS database instance, as in the following diagram.
In this example, the application is responsible for handling and persisting the order data, as well as dealing with increases in traffic for popular items.
One potential point of vulnerability in the order processing workflow is in saving the order in the database. The business expects that every order has been persisted into the database. However, any potential deadlock, race condition, or network issue could cause the persistence of the order to fail. Then, the order is lost with no recourse to restore the order.
With good logging capability, you may be able to identify when an error occurred and which customer order failed. This wouldn’t allow you to “restore” the transaction, and by that stage, your customer is no longer your customer.
As illustrated in the following diagram, introducing an SQS queue helps improve your ordering application. Using the queue isolates the processing logic into its own component and runs it in a separate process from the web application. This, in turn, allows the system to be more resilient to spikes in traffic, while allowing work to be performed only as fast as necessary in order to manage costs.
In addition, you now have a mechanism for persisting orders as messages (with the queue acting as a temporary database), and have moved the scope of your transaction with your database further down the stack. In the event of an application exception or transaction failure, this ensures that the order processing can be retired or redirected to the Amazon SQS Dead Letter Queue (DLQ), for re-processing at a later stage. (See the recent post, Using Amazon SQS Dead-Letter Queues to Control Message Failure, for more information on dead-letter queues.)
Scaling the order processing nodes
This change allows you now to scale the web application frontend independently from the processing nodes. The frontend application can continue to scale based on metrics such as CPU usage, or the number of requests hitting the load balancer. Processing nodes can scale based on the number of orders in the queue. Here is an example of scale-in and scale-out alarms that you would associate with the scaling policy.
Scale-out Alarm
Scaling the order processing implementation
On top of scaling at an infrastructure level using Auto Scaling, make sure to take advantage of the processing power of your Amazon EC2 instances by using as many of the available threads as possible. There are several ways to implement this. In this post, we build a Windows service that uses the BackgroundWorker class to process the messages from the queue.
Here’s a closer look at the implementation. In the first section of the consuming application, use a loop to continually poll the queue for new messages, and construct a ReceiveMessageRequest variable.
public static void PollQueue()
{
while (_running)
{
Task<ReceiveMessageResponse> receiveMessageResponse;
// Pull messages off the queue
using (var sqs = new AmazonSQSClient())
{
const int maxMessages = 10; // 1-10
//Receiving a message
var receiveMessageRequest = new ReceiveMessageRequest
{
// Get URL from Configuration
QueueUrl = _queueUrl,
// The maximum number of messages to return.
// Fewer messages might be returned.
MaxNumberOfMessages = maxMessages,
// A list of attributes that need to be returned with message.
AttributeNames = new List<string> { "All" },
// Enable long polling.
// Time to wait for message to arrive on queue.
WaitTimeSeconds = 5
};
receiveMessageResponse = sqs.ReceiveMessageAsync(receiveMessageRequest);
}
The WaitTimeSeconds property of the ReceiveMessageRequest specifies the duration (in seconds) that the call waits for a message to arrive in the queue before returning a response to the calling application. There are a few benefits to using long polling:
- It reduces the number of empty responses by allowing SQS to wait until a message is available in the queue before sending a response.
- It eliminates false empty responses by querying all (rather than a limited number) of the servers.
- It returns messages as soon any message becomes available.
For more information, see Amazon SQS Long Polling.
After you have returned messages from the queue, you can start to process them by looping through each message in the response and invoking a new BackgroundWorker thread.
// Process messages
if (receiveMessageResponse.Result.Messages != null)
{
foreach (var message in receiveMessageResponse.Result.Messages)
{
Console.WriteLine("Received SQS message, starting worker thread");
// Create background worker to process message
BackgroundWorker worker = new BackgroundWorker();
worker.DoWork += (obj, e) => ProcessMessage(message);
worker.RunWorkerAsync();
}
}
else
{
Console.WriteLine("No messages on queue");
}
The event handler, ProcessMessage, is where you implement business logic for processing orders. It is important to have a good understanding of how long a typical transaction takes so you can set a message VisibilityTimeout that is long enough to complete your operation. If order processing takes longer than the specified timeout period, the message becomes visible on the queue. Other nodes may pick it and process the same order twice, leading to unintended consequences.
Handling Duplicate Messages
In order to manage duplicate messages, seek to make your processing application idempotent. In mathematics, idempotent describes a function that produces the same result if it is applied to itself:
f(x) = f(f(x))
No matter how many times you process the same message, the end result is the same (definition from Enterprise Integration Patterns: Designing, Building, and Deploying Messaging Solutions, Hohpe and Wolf, 2004).
There are several strategies you could apply to achieve this:
- Create messages that have inherent idempotent characteristics. That is, they are non-transactional in nature and are unique at a specified point in time. Rather than saying “place new order for Customer A,” which adds a duplicate order to the customer, use “place order <orderid> on <timestamp> for Customer A,” which creates a single order no matter how often it is persisted.
- Deliver your messages via an Amazon SQS FIFO queue, which provides the benefits of message sequencing, but also mechanisms for content-based deduplication. You can deduplicate using the MessageDeduplicationId property on the SendMessage request or by enabling content-based deduplication on the queue, which generates a hash for MessageDeduplicationId, based on the content of the message, not the attributes.
var sendMessageRequest = new SendMessageRequest
{
QueueUrl = _queueUrl,
MessageBody = JsonConvert.SerializeObject(order),
MessageGroupId = Guid.NewGuid().ToString("N"),
MessageDeduplicationId = Guid.NewGuid().ToString("N")
};
- If using SQS FIFO queues is not an option, keep a message log of all messages attributes processed for a specified period of time, as an alternative to message deduplication on the receiving end. Verifying the existence of the message in the log before processing the message adds additional computational overhead to your processing. This can be minimized through low latency persistence solutions such as Amazon DynamoDB. Bear in mind that this solution is dependent on the successful, distributed transaction of the message and the message log.
Handling exceptions
Because of the distributed nature of SQS queues, it does not automatically delete the message. Therefore, you must explicitly delete the message from the queue after processing it, using the message ReceiptHandle property (see the following code example).
However, if at any stage you have an exception, avoid handling it as you normally would. The intention is to make sure that the message ends back on the queue, so that you can gracefully deal with intermittent failures. Instead, log the exception to capture diagnostic information, and swallow it.
By not explicitly deleting the message from the queue, you can take advantage of the VisibilityTimeout behavior described earlier. Gracefully handle the message processing failure and make the unprocessed message available to other nodes to process.
In the event that subsequent retries fail, SQS automatically moves the message to the configured DLQ after the configured number of receives has been reached. You can further investigate why the order process failed. Most importantly, the order has not been lost, and your customer is still your customer.
private static void ProcessMessage(Message message)
{
using (var sqs = new AmazonSQSClient())
{
try
{
Console.WriteLine("Processing message id: {0}", message.MessageId);
// Implement messaging processing here
// Ensure no downstream resource contention (parallel processing)
// <your order processing logic in here…>
Console.WriteLine("{0} Thread {1}: {2}", DateTime.Now.ToString("s"), Thread.CurrentThread.ManagedThreadId, message.MessageId);
// Delete the message off the queue.
// Receipt handle is the identifier you must provide
// when deleting the message.
var deleteRequest = new DeleteMessageRequest(_queueName, message.ReceiptHandle);
sqs.DeleteMessageAsync(deleteRequest);
Console.WriteLine("Processed message id: {0}", message.MessageId);
}
catch (Exception ex)
{
// Do nothing.
// Swallow exception, message will return to the queue when
// visibility timeout has been exceeded.
Console.WriteLine("Could not process message due to error. Exception: {0}", ex.Message);
}
}
}
Using SQS to adapt to changing business requirements
One of the benefits of introducing a message queue is that you can accommodate new business requirements without dramatically affecting your application.
If, for example, the business decided that all orders placed over $5000 are to be handled as a priority, you could introduce a new “priority order” queue. The way the orders are processed does not change. The only significant change to the processing application is to ensure that messages from the “priority order” queue are processed before the “standard order” queue.
The following diagram shows how this logic could be isolated in an “order dispatcher,” whose only purpose is to route order messages to the appropriate queue based on whether the order exceeds $5000. Nothing on the web application or the processing nodes changes other than the target queue to which the order is sent. The rates at which orders are processed can be achieved by modifying the poll rates and scalability settings that I have already discussed.
Extending the design pattern with Amazon SNS
Amazon SNS supports reliable publish-subscribe (pub-sub) scenarios and push notifications to known endpoints across a wide variety of protocols. It eliminates the need to periodically check or poll for new information and updates. SNS supports:
- Reliable storage of messages for immediate or delayed processing
- Publish / subscribe – direct, broadcast, targeted “push” messaging
- Multiple subscriber protocols
- Amazon SQS, HTTP, HTTPS, email, SMS, mobile push, AWS Lambda
With these capabilities, you can provide parallel asynchronous processing of orders in the system and extend it to support any number of different business use cases without affecting the production environment. This is commonly referred to as a “fanout” scenario.
Rather than your web application pushing orders to a queue for processing, send a notification via SNS. The SNS messages are sent to a topic and then replicated and pushed to multiple SQS queues and Lambda functions for processing.
As the diagram above shows, you have the development team consuming “live” data as they work on the next version of the processing application, or potentially using the messages to troubleshoot issues in production.
Marketing is consuming all order information, via a Lambda function that has subscribed to the SNS topic, inserting the records into an Amazon Redshift warehouse for analysis.
All of this, of course, is happening without affecting your order processing application.
Summary
While I haven’t dived deep into the specifics of each service, I have discussed how these services can be applied at an architectural level to build loosely coupled systems that facilitate multiple business use cases. I’ve also shown you how to use infrastructure and application-level scaling techniques, so you can get the most out of your EC2 instances.
One of the many benefits of using these managed services is how quickly and easily you can implement powerful messaging capabilities in your systems, and lower the capital and operational costs of managing your own messaging middleware.
Using Amazon SQS and Amazon SNS together can provide you with a powerful mechanism for decoupling application components. This should be part of design considerations as you architect for the cloud.
For more information, see the Amazon SQS Developer Guide and Amazon SNS Developer Guide. You’ll find tutorials on all the concepts covered in this post, and more. To can get started using the AWS console or SDK of your choice visit:
Happy messaging!