Networking & Content Delivery
Using the AWS CDK and AWS Transit Gateway Inter-Region peering to build a global network
An Amazon VPC is a logically isolated section of the AWS cloud. Some of our largest enterprise customers have global networks containing VPCs that need to communicate across different AWS Regions, even across different AWS accounts. While this can appear like a cumbersome and complex task, with AWS Transit Gateway Inter-Region peering, it can be greatly simplified – especially when combined with the power of the AWS Cloud Development Kit (CDK).
In this blog post, you’ll go through the creation of a global network that spans multiple AWS Regions using AWS Transit Gateway Inter-Region peering. You will also go over key considerations and take a look at the solution overview, followed by configuration walkthroughs using the CDK. The AWS CDK is an open source software development framework to model and provision your cloud application resources using familiar programming languages, such as Python, which is what we’re using in this blog post.
AWS Transit Gateway is a service that lets you connect your VPCs and your on-premises networks. Transit gateway inter-region peering became generally available during re:Invent 2019 and it’s now easy to connect transit gateways across multiple AWS Regions that are deployed within the same, or in different, AWS accounts.
Considerations
When creating a global network with transit gateway, consider these network design principles:
- Ensure that your VPC network ranges (CIDR blocks) do not overlap.
- Make sure the solution you choose is able to scale according to your current and future VPC connectivity needs.
- Ensure you implement a highly available (HA) design with no single point of failure.
- Avoid building in regional dependencies to application architectures.
- With transit gateway, you are charged for the number of connections that you make to the transit gateway per hour and for the amount of traffic that flows through the transit gateway. See AWS Transit Gateway pricing for details.
- Take quotas for your transit gateways into consideration.
Solution overview
The following diagram will be referred to throughout this blog post:
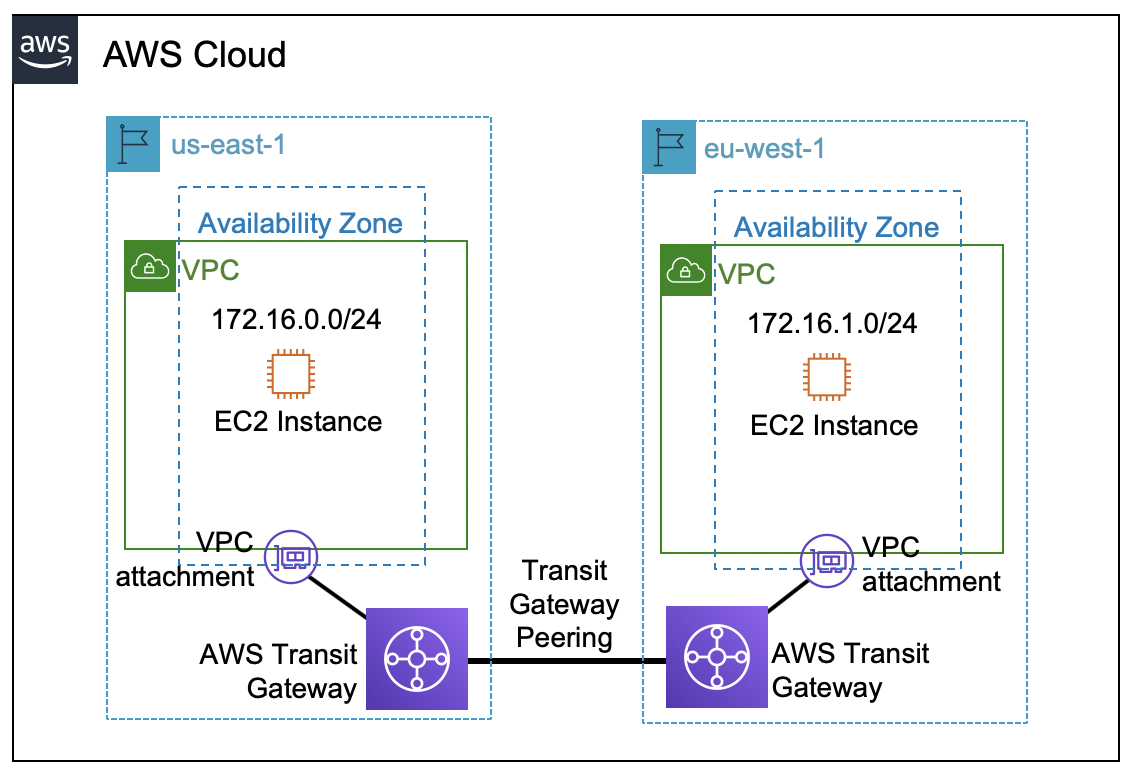
Figure 1 – Solution diagram for this blog post
While the transit gateway only connects to VPCs within the same Region, you can establish peering connections between AWS Transit Gateways in different AWS Regions. This lets you build global, cloud-based networks. Traffic using transit gateway peering stays on the AWS global network, never traverses the public internet and is encrypted in flight – so it always takes the most optimal path, in the most secure way.
By following these steps, you will also launch one EC2 instance in two separate Regions, as well as VPC endpoints to access the instances using AWS Systems Manager Session Manager. This allows you to verify two-way connectivity by pinging from one instance to the other.
Deployment steps
Pre-requisites:
- An AWS account without any existing transit gateways in us-east-1 or eu-west-1
- AWS CLI, authenticated and configured
- Python 3.6+
- AWS CDK
- Git
Step 1: Using your device’s command line, check out our Git repository to a local directory on your device:
git clone https://github.com/aws-samples/aws-cdk-transit-gateway-peering
Step 2: Change directories to the new directory that was created during the previous step:
cd aws-cdk-transit-gateway-peering/
Step 2a: Copy the following JSON document to tell the AWS CDK which command to use to run your app. (This is for Windows only):
Windows: copy cdk-windows.json cdk.json /Y
Step 3: Create a virtual environment:
macOS/Linux: python3 -m venv .env
Windows: python -m venv .env
Step 4: Activate the virtual environment after the init process completes and the virtual environment is created:
macOS/Linux: source .env/bin/activate
Windows: .env\Scripts\activate.bat
Step 5: Install the required dependencies:
pip3 install -r requirements.txt
Step 6: Synthesize the templates. AWS CDK apps use code to define the infrastructure, and when run, they produce, or “synthesize” an AWS CloudFormation template for each stack defined in the application:
cdk synthesize
Step 7: Deploy the solution. By default, some actions that could potentially make security changes, require approval. In this deployment, you are creating an IAM role for the EC2 instances and creating security groups. The following command overrides the approval prompts but if you would like to manually accept the prompts, omit the “--require-approval never
” flag:
cdk deploy "*" --require-approval never
While the AWS CDK deploys the CloudFormation stacks, you can follow the deployment progress in your terminal:
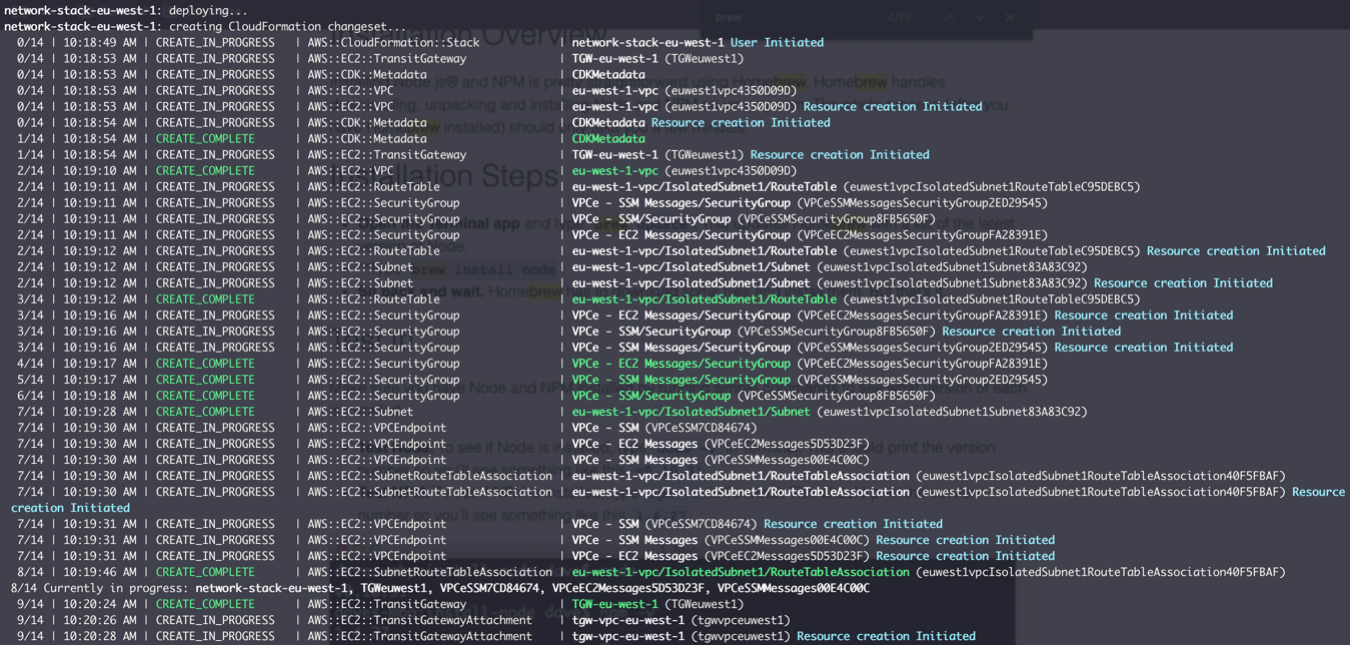
Figure 2 – deployment process
The code in the GitHub project deploys resources in us-east-1 and eu-west-1, including VPCs, transit gateways, VPC endpoints, and EC2 instances.
The deployment is divided into four stacks, two per Region. Within each Region, the first stack deploys the network and the second stack deploys the EC2 instance. There is an explicit dependency between the stacks to ensure that the underlying network infrastructure exists before you create the EC2 instances.
The relevant code in the app.py
file is shown below:
network_stack_us_east_1 = Network(app, "network-stack-us-east-1",
cidr_range="172.16.0.0/24",
tgw_asn=64512,
env={
'region': 'us-east-1',
}
)
network_stack_eu_west_1 = Network(app, "network-stack-eu-west-1",
cidr_range="172.16.1.0/24",
tgw_asn=64513,
env={
'region': 'eu-west-1',
}
)
ec2_stack_us_east_1 = Ec2(app, id="instance-stack-us-east-1",
network_stack=network_stack_us_east_1,
env={
'region': 'us-east-1',
}
)
ec2_stack_eu_west_1 = Ec2(app, id="instance-stack-eu-west-1",
network_stack=network_stack_eu_west_1,
env={
'region': 'eu-west-1',
}
)
ec2_stack_us_east_1.add_dependency(network_stack_us_east_1)
ec2_stack_eu_west_1.add_dependency(network_stack_eu_west_1)
Step 8: Once the stacks have successfully deployed, execute the series of Python scripts that you checked out from the Git repository during step 1. Python scripts are required as transit gateway peering is not yet natively supported by AWS CloudFormation. Establish the transit gateway peering connection:
macOS/Linux: python3 create-tgw-peering.py
Windows: python create-tgw-peering.py
Initially, the peering connection’s state change shows as “initiatingRequest” – but it should only remain that way for less than a minute. Run the following verification command and validate, in the output, that the peering connection’s state is showing as “pendingAcceptance” before proceeding to step 9:
aws ec2 describe-transit-gateway-peering-attachments --region us-east-1
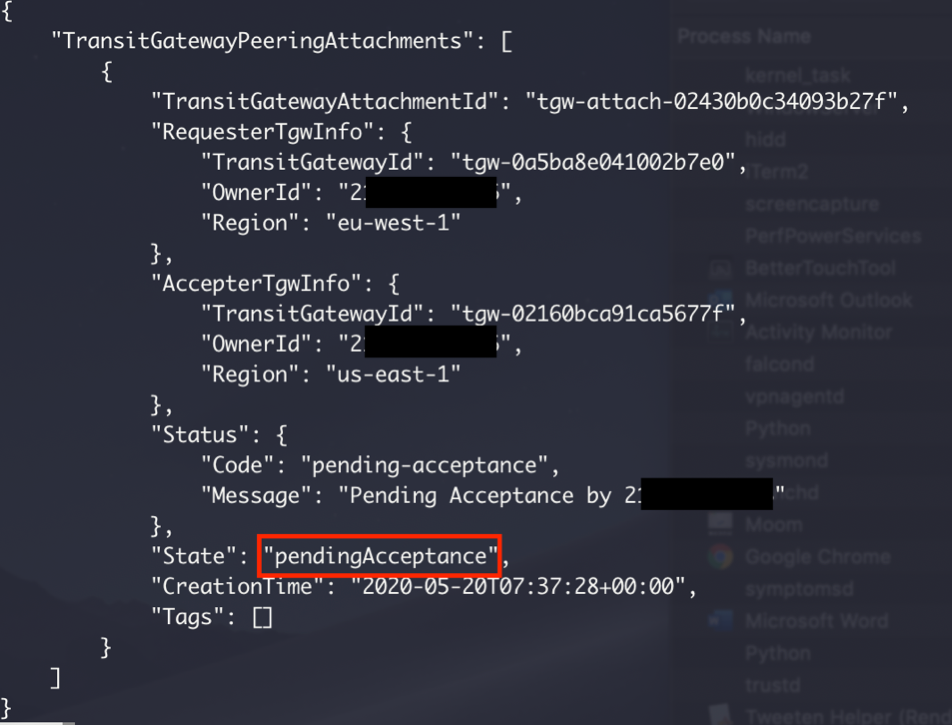
Figure 3 – output of describe-transit-gateway-peering-attachments command
Step 9: Accept the peering request:
macOS/Linux: python3 accept-tgw-peering.py
Windows: python accept-tgw-peering.py
Shortly after accepting the peering request, the peering connection’s state will show as “pending” and it will remain in that state for a few minutes. Run the following verification command and ensure that, in the output, the peering connection’s state is showing as “available” before moving on to step 10:
aws ec2 describe-transit-gateway-peering-attachments --region us-east-1
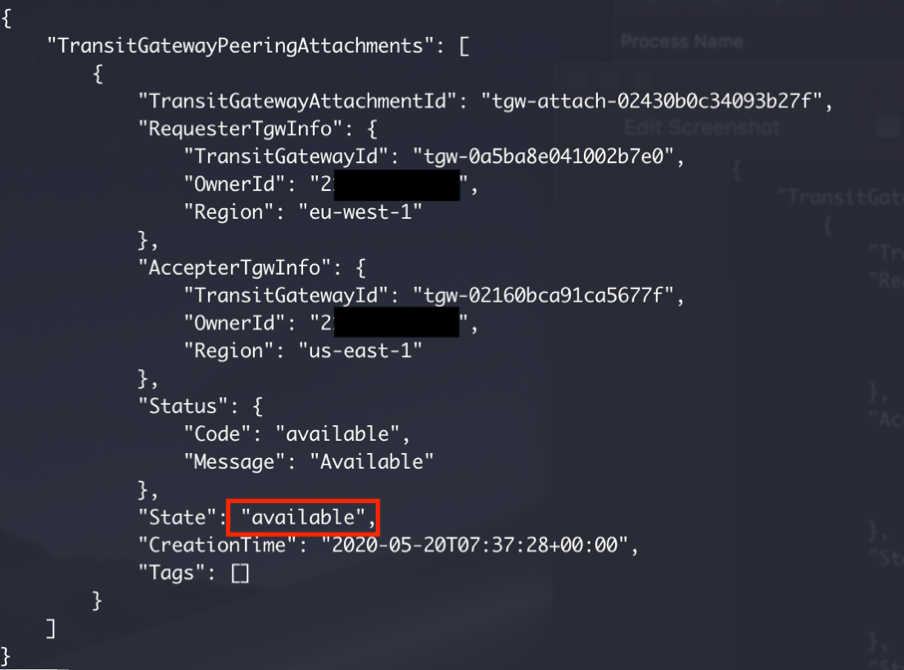
Figure 4 – output of validation command
Step 10: Once the peering connection has changed to “available”, add a route to each AWS Transit Gateway’s route table:
macOS/Linux: python3 create-tgw-routes.py
Windows: python create-tgw-routes.py
Verification steps
To verify cross-region network connectivity, log into the AWS Management Console, select the us-east-1 Region and navigate to the EC2 service. Select “running instances” and then select the EC2 instance that was created during step 7 of the deployment procedure when the AWS CDK deployed the stacks. Scroll down and take note of the private IP address. Also, take note of the private IP address for the EC2 instance in the eu-west-1 Region.
Select the EC2 instance in either Region and choose Connect. For the connection method, select Session Manager and click on Connect:
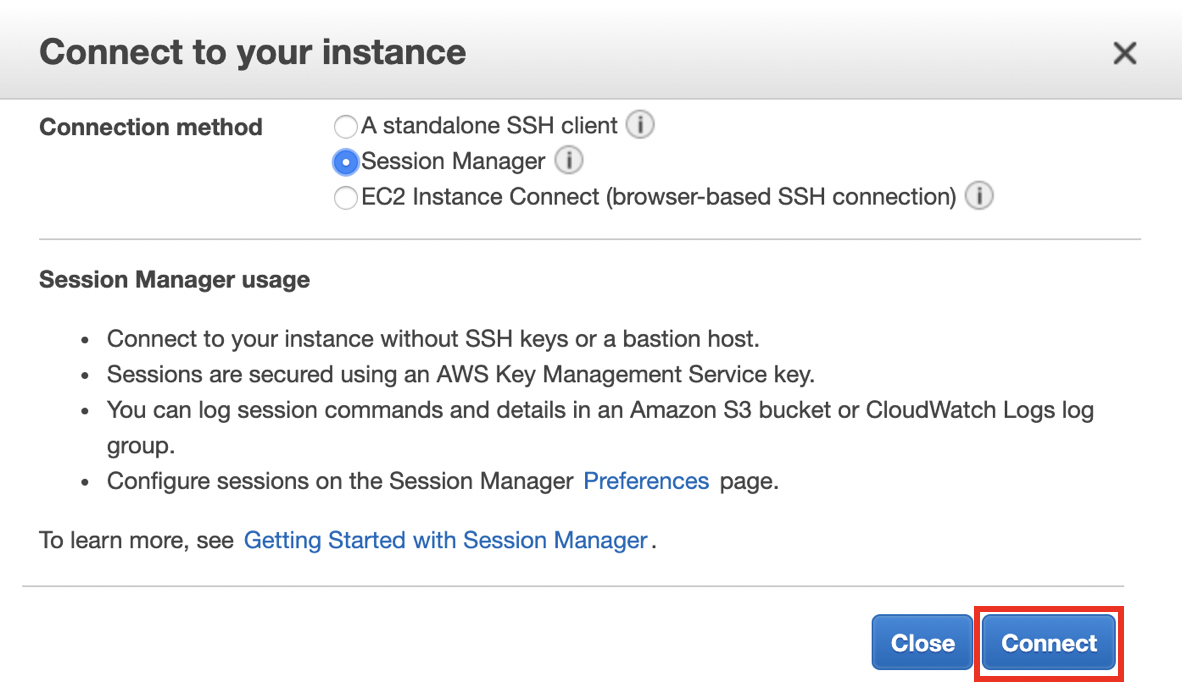
Figure 5 – connect to your instance using Session Manager
Ping the private IP address of the EC2 instance in the opposite Region in order to confirm end-to-end network connectivity:
ping [private IP address of EC2 instance in opposite Region]
If the ping packets are transmitted and received as in the next screenshot, congratulations! You’ve properly enabled transit gateway peering and validated end to end IP connectivity.
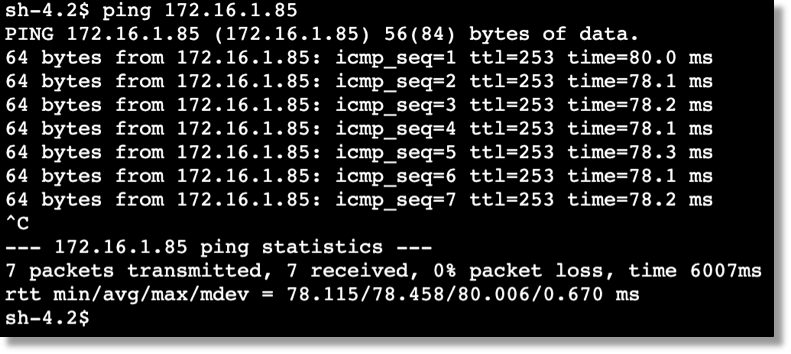
Figure 6 – successful ping test
If you are not receiving pings, go through the previous steps to ensure that you haven’t missed anything or made any misconfigurations.
Cleanup
Follow these steps to remove the resources that were deployed in this post.
Step 1: Delete the two transit gateway routes that were created to send traffic across the peering connection and also delete the peering connection itself:
macOS/Linux: python3 cleanup.py
Windows: python cleanup.py
It takes a few minutes for the peering connection to be deleted. Run the following command and ensure that, in the output, the peering connection’s state is showing as “deleted” before moving on to step 2:
aws ec2 describe-transit-gateway-peering-attachments --region us-east-1
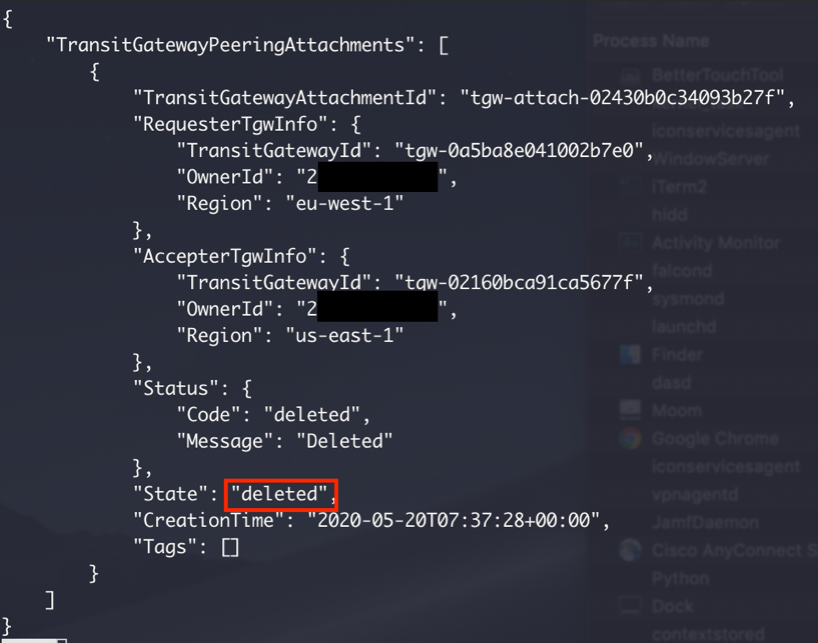
Figure 7 – output of describe-transit-gateway-peering-attachments command
Step 2: Terminate the rest of the resources with the following command:
cdk destroy "*"
When asked to confirm the deletion of the four stacks, select “y
”.
Conclusion
By following this blog post, you became more familiar with how to use AWS Transit Gateway Inter-Region peering to create a network spanning two AWS Regions. You also learned how to automate the creation of the resources using the AWS CDK and modeled your cloud resources using Python.
You can scale this functionality to peer a larger quantity of AWS Transit Gateways, each connecting to many VPCs, across many Regions. Truly, AWS Transit Gateway Inter-Region peering allows you to scale your global network – by keeping things simple!
As a next step, check out the AWS blog post that discusses Transit Gateway Network Manager, enabling you to centrally manage your networks that are built around transit gateways.