AWS Security Blog
How to customize access tokens in Amazon Cognito user pools
With Amazon Cognito, you can implement customer identity and access management (CIAM) into your web and mobile applications. You can add user authentication and access control to your applications in minutes.
In this post, I introduce you to the new access token customization feature for Amazon Cognito user pools and show you how to use it. Access token customization is included in the advanced security features (ASF) of Amazon Cognito. Note that ASF is subject to additional pricing as described on the Amazon Cognito pricing page.
What is access token customization?
When a user signs in to your app, Amazon Cognito verifies their sign-in information, and if the user is authenticated successfully, returns the ID, access, and refresh tokens. The access token, which uses the JSON Web Token (JWT) format following the RFC7519 standard, contains claims in the token payload that identify the principal being authenticated, and session attributes such as authentication time and token expiration time. More importantly, the access token also contains authorization attributes in the form of user group memberships and OAuth scopes. Your applications or API resource servers can evaluate the token claims to authorize specific actions on behalf of users.
With access token customization, you can add application-specific claims to the standard access token and then make fine-grained authorization decisions to provide a differentiated end-user experience. You can refine the original scope claims to further restrict access to your resources and enforce the least privileged access. You can also enrich access tokens with claims from other sources, such as user subscription information stored in an Amazon DynamoDB table. Your application can use this enriched claim to determine the level of access and content available to the user. This reduces the need to build a custom solution to look up attributes in your application’s code, thereby reducing application complexity, improving performance, and smoothing the integration experience with downstream applications.
How do I use the access token customization feature?
Amazon Cognito works with AWS Lambda functions to modify your user pool’s authentication behavior and end-user experience. In this section, you’ll learn how to configure a pre token generation Lambda trigger function and invoke it during the Amazon Cognito authentication process. I’ll also show you an example function to help you write your own Lambda function.
Lambda trigger flow
During a user authentication, you can choose to have Amazon Cognito invoke a pre token generation trigger to enrich and customize your tokens.
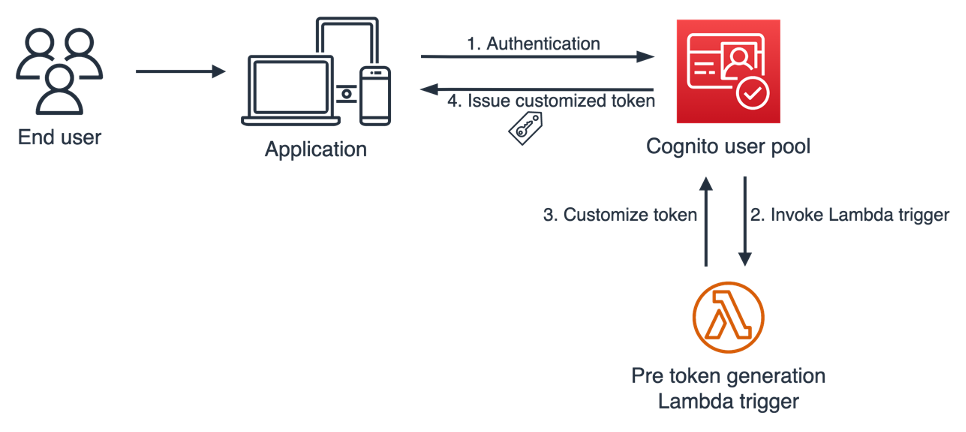
Figure 1: Pre token generation trigger flow
Figure 1 illustrates the pre token generation trigger flow. This flow has the following steps:
- An end user signs in to your app and authenticates with an Amazon Cognito user pool.
- After the user completes the authentication, Amazon Cognito invokes the pre token generation Lambda trigger, and sends event data to your Lambda function, such as userAttributes and scopes, in a pre token generation trigger event.
- Your Lambda function code processes token enrichment logic, and returns a response event to Amazon Cognito to indicate the claims that you want to add or suppress.
- Amazon Cognito vends a customized JWT to your application.
The pre token generation trigger flow supports OAuth 2.0 grant types, such as the authorization code grant flow and implicit grant flow, and also supports user authentication through the AWS SDK.
Enable access token customization
Your Amazon Cognito user pool delivers two different versions of the pre token generation trigger event to your Lambda function. Trigger event version 1 includes userAttributes, groupConfiguration, and clientMetadata in the event request, which you can use to customize ID token claims. Trigger event version 2 adds scope in the event request, which you can use to customize scopes in the access token in addition to customizing other claims.
In this section, I’ll show you how to update your user pool to trigger event version 2 and enable access token customization.
To enable access token customization
- Open the Cognito user pool console, and then choose User pools.
- Choose the target user pool for token customization.
- On the User pool properties tab, in the Lambda triggers section, choose Add Lambda trigger.
- In the Lambda triggers section, do the following:
- For Trigger type, select Authentication.
- For Authentication, select Pre token generation trigger.
- For Trigger event version, select Basic features + access token customization – Recommended. If this option isn’t available to you, make sure that you have enabled advanced security features. You must have advanced security features enabled to access this option.
- Select your Lambda function and assign it as the pre token generation trigger. Then choose Add Lambda trigger.
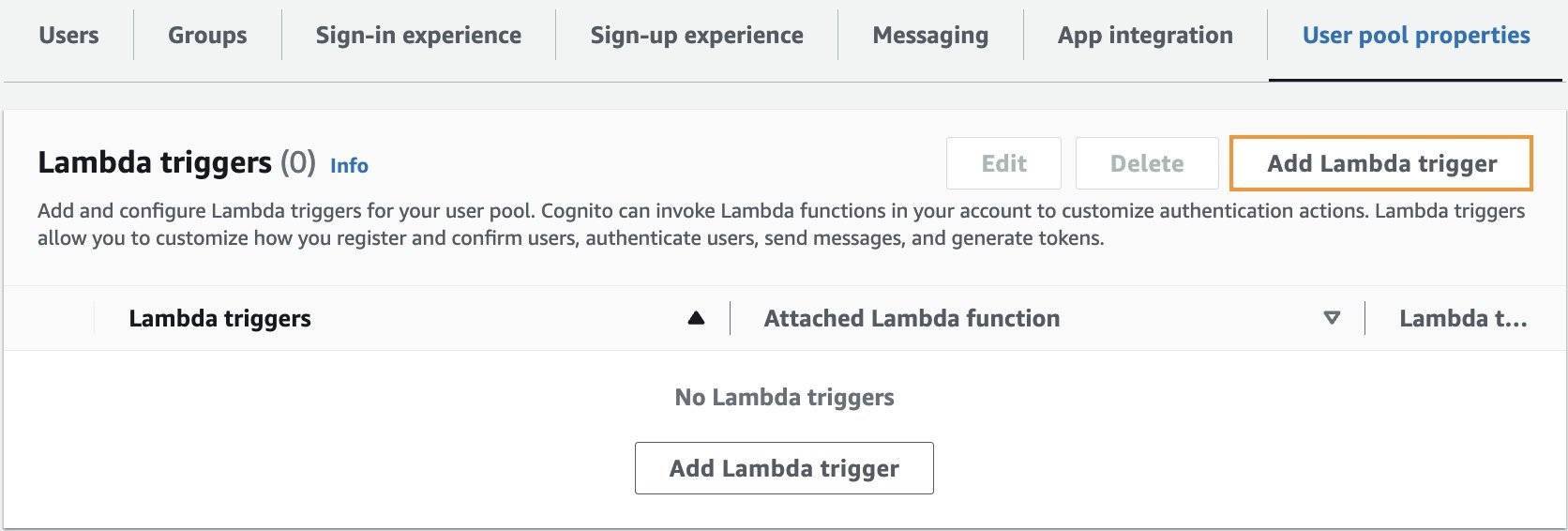
Figure 2: Add Lambda trigger
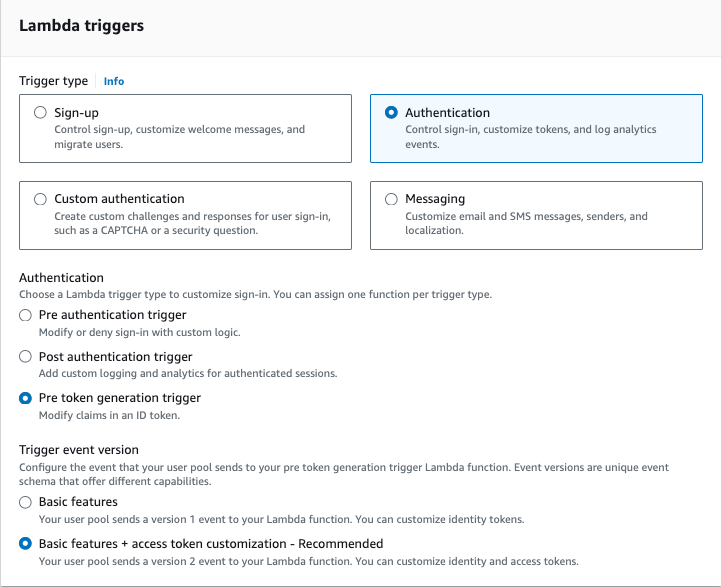
Figure 3: Select Lambda trigger
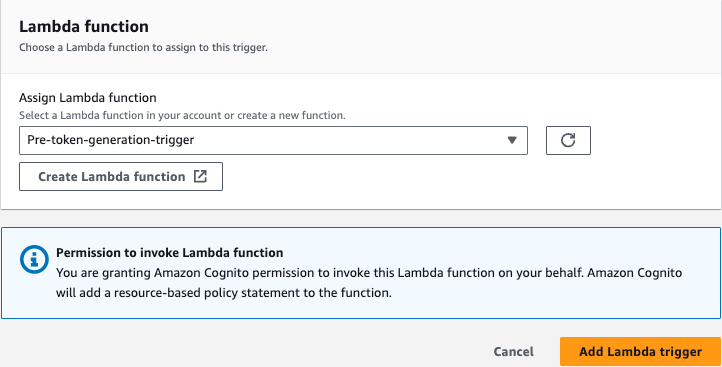
Figure 4: Add Lambda trigger
Example pre token generation trigger
Now that you have enabled access token customization, I’ll walk you through a code example of the pre token generation Lambda trigger, and the version 2 trigger event. This code example examines the trigger event request, and adds a new custom claim and a custom OAuth scope in the response for Amazon Cognito to customize the access token to suit various authorization scheme.
Here is an example version 2 trigger event. The event request contains the user attributes from the Amazon Cognito user pool, the original scope claims, and the original group configurations. It has two custom attributes—membership and location—which are collected during the user registration process and stored in the Cognito user pool.
{
"version": "2",
"triggerSource": "TokenGeneration_HostedAuth",
"region": "us-east-1",
"userPoolId": "us-east-1_01EXAMPLE",
"userName": "mytestuser",
"callerContext": {
"awsSdkVersion": "aws-sdk-unknown-unknown",
"clientId": "1example23456789"
},
"request": {
"userAttributes": {
"sub": "a1b2c3d4-5678-90ab-cdef-EXAMPLE11111",
"cognito:user_status": "CONFIRMED",
"email": "my-test-user@example.com",
"email_verified": "true",
"custom:membership": "Premium",
"custom:location": "USA"
},
"groupConfiguration": {
"groupsToOverride": [],
"iamRolesToOverride": [],
"preferredRole": null
},
"scopes": [
"openid",
"profile",
"email"
]
},
"response": {
"claimsAndScopeOverrideDetails": null
}
}
In the following code example, I transformed the user’s location attribute and membership attribute to add a custom claim and a custom scope. I used the claimsToAddOrOverride field to create a new custom claim called demo:membershipLevel with a membership value of Premium from the event request. I also constructed a new scope with the value of membership:USA.Premium through the scopesToAdd claim, and added the new claim and scope in the event response.
export const handler = function(event, context) {
// Retrieve user attribute from event request
const userAttributes = event.request.userAttributes;
// Add scope to event response
event.response = {
"claimsAndScopeOverrideDetails": {
"idTokenGeneration": {},
"accessTokenGeneration": {
"claimsToAddOrOverride": {
"demo:membershipLevel": userAttributes['custom:membership']
},
"scopesToAdd": ["membership:" + userAttributes['custom:location'] + "." + userAttributes['custom:membership']]
}
}
};
// Return to Amazon Cognito
context.done(null, event);
};
With the preceding code, the Lambda trigger sends the following response back to Amazon Cognito to indicate the customization that was needed for the access tokens.
"response": {
"claimsAndScopeOverrideDetails": {
"idTokenGeneration": {},
"accessTokenGeneration": {
"claimsToAddOrOverride": {
"demo:membershipLevel": "Premium"
},
"scopesToAdd": [
"membership:USA.Premium"
]
}
}
}
Then Amazon Cognito issues tokens with these customizations at runtime:
{
"sub": "a1b2c3d4-5678-90ab-cdef-EXAMPLE11111",
"iss": "https://cognito-idp.us-east-1.amazonaws.com/us-east-1_01EXAMPLE",
"version": 2,
"client_id": "1example23456789",
"event_id": "01faa385-562d-4730-8c3b-458e5c8f537b",
"token_use": "access",
"demo:membershipLevel": "Premium",
"scope": "openid profile email membership:USA.Premium",
"auth_time": 1702270800,
"exp": 1702271100,
"iat": 1702270800,
"jti": "d903dcdf-8c73-45e3-bf44-51bf7c395e06",
"username": "mytestuser"
}
Your application can then use the newly-minted, custom scope and claim to authorize users and provide them with a personalized experience.
Considerations and best practices
There are four general considerations and best practices that you can follow:
- Some claims and scopes aren’t customizable. For example, you can’t customize claims such as auth_time, iss, and sub, or scopes such as aws.cognito.signin.user.admin. For the full list of excluded claims and scopes, see the Excluded claims and scopes.
- Work backwards from authorization. When you customize access tokens, you should start with your existing authorization schema and then decide whether to customize the scopes or claims, or both. Standard OAuth based authorization scenarios, such as Amazon API Gateway authorizers, typically use custom scopes to provide access. However, if you have complex or fine-grained authorization requirements, then you should consider using both scopes and custom claims to pass additional contextual data to the application or to a policy-based access control service such as Amazon Verified Permissions.
- Establish governance in token customization. You should have a consistent company engineering policy to provide nomenclature guidance for scopes and claims. A syntax standard promotes globally unique variables and avoids a name collision across different application teams. For example, Application X at AnyCompany can choose to name their scope as ac.appx.claim_name, where ac represents AnyCompany as a global identifier and appx.claim_name represents Application X’s custom claim.
- Be aware of limits. Because tokens are passed through various networks and systems, you need to be aware of potential token size limitations in your systems. You should keep scope and claim names as short as possible, while still being descriptive.
Conclusion
In this post, you learned how to integrate a pre token generation Lambda trigger with your Amazon Cognito user pool to customize access tokens. You can use the access token customization feature to provide differentiated services to your end users based on claims and OAuth scopes. For more information, see pre token generation Lambda trigger in the Amazon Cognito Developer Guide.
If you have feedback about this post, submit comments in the Comments section below. If you have questions about this post, contact AWS Support.
Want more AWS Security news? Follow us on Twitter.