AWS Compute Blog
Building an automated knowledge repo with Amazon EventBridge and Zendesk
Zendesk Guide is a smart knowledge base that helps customers harness the power of institutional knowledge. It enables users to build a customizable help center and customer portal.
This post shows how to implement a bidirectional event orchestration pattern between AWS services and an Amazon EventBridge third-party integration partner. This example uses support ticket events to build a customer self-service knowledge repository. It uses the EventBridge partner integration with Zendesk to accelerate the growth of a customer help center.
The examples in this post are part of a serverless application called FreshTracks. This is built in Vue.js and demonstrates SaaS integrations with Amazon EventBridge. To test this example, ask a question on the Fresh Tracks application.
The backend components for this EventBridge integration with Zendesk have been extracted into a separate example application in this GitHub repo.
How the application works
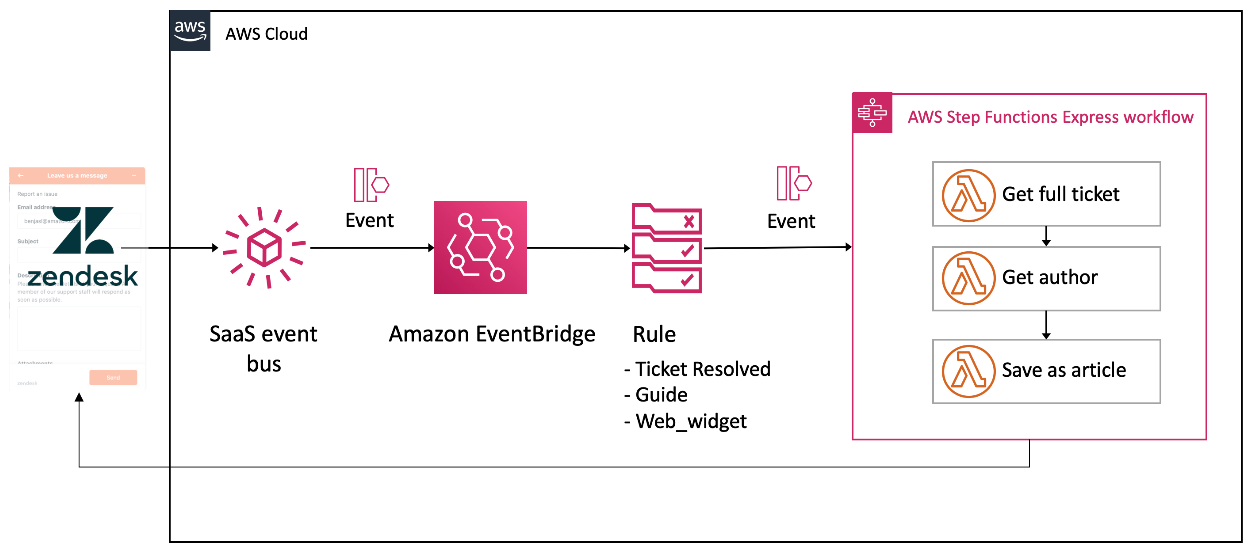
Routing Zendesk events with Amazon EventBridge.
- A user searches the knowledge repository via a widget embedded in the web application.
- If there is no answer, the user submits the question via the web widget.
- Zendesk receives the question as a support ticket.
- Zendesk emits events when the support ticket is resolved.
- These events are streamed into a custom SaaS event bus in EventBridge.
- Event rules match events and send them downstream to an AWS Step Functions Express Workflow.
- The Express Workflow orchestrates Lambda functions to retrieve additional information about the event with the Zendesk API.
- A Lambda function uses the Zendesk API to publish a new help article from the support ticket data.
- The new article is searchable on the website widget for other users to read.
Before deploying this application, you must generate an API key from within Zendesk.
Creating the Zendesk API resource
Use an API to execute events on your Zendesk account from AWS. Follow these steps to generate a Zendesk API token. This is used by the application to authenticate Zendesk API calls.
To generate an API token
- Log in to the Zendesk dashboard.
- Click the Admin icon in the sidebar, then select Channels > API.
- Click the Settings tab, and make sure that Token Access is enabled.
- Click the + button to the right of Active API Tokens.
Creating a Zendesk API token.
- Copy the token, and store it securely. Once you close this window, the full token is not displayed again.
- Click Save to return to the API page, which shows a truncated version of the token.
Zendesk API token.
Configuring Zendesk with Amazon EventBridge
Step 1. Configuring your Zendesk event source.
- Go to your Zendesk Admin Center and select Admin Center > Integrations.
- Choose Connect in events Connector for Amazon EventBridge to open the page to configure your Zendesk event source.
Zendesk integrations
- Enter your AWS account ID in the Amazon Web Services account ID field, and select the Region to receive events.
- Choose Save.
Zendesk Amazon EventBridge configuration.
Step 2. Associate the Zendesk event source with a new event bus:
- Log into the AWS Management Console and navigate to services > Amazon EventBridge > Partner event sources
New event source
- Select the radio button next to the new event source and choose Associate with event bus.
Associating event source with event bus.
- Choose Associate.
Deploying the backend application
After associating the Event source with a new partner event bus, you can deploy backend services to receive events.
To set up the example application, visit the GitHub repo and follow the instructions in the README.md file.
When deploying the application stack, make sure to provide the custom event bus name, and Zendesk API credentials with --parameter-overrides
.
sam deploy --parameter-overrides ZendeskEventBusName=aws.partner/zendesk.com/123456789/default ZenDeskDomain=MydendeskDomain ZenDeskPassword=myAPITOken ZenDeskUsername=myZendeskAgentUsername
You can find the name of the new Zendesk custom event bus in the custom event bus section of the EventBridge console.
Routing events with rules
When a support ticket is updated in Zendesk, a number of individual events are streamed to EventBridge, these include an event for each of:
- Agent Assignment Changed
- Comment Created
- Status Changed
- Brand Changed
- Subject Changed
An EventBridge rule is used filter for events. The AWS Serverless Application Model (SAM) template defines the rule with the `AWS::Events::Rule` resource type. This routes the event downstream to an AWS Step Functions Express Workflow. The EventPattern is shown below:
ZendeskNewWebQueryClosed:
Type: AWS::Events::Rule
Properties:
Description: "New Web Query"
EventBusName:
Ref: ZendeskEventBusName
EventPattern:
account:
- !Sub '${AWS::AccountId}'
detail-type:
- "Support Ticket: Comment Created"
detail:
ticket_event:
ticket:
status:
- solved
tags:
- web_widget
tags:
- guide
Targets:
- RoleArn: !GetAtt [ MyStatesExecutionRole, Arn ]
Arn: !Ref FreshTracksZenDeskQueryMachine
Id: NewQuery
The tickets must have two specific tags (web_widget and guide) for this pattern to match. These are defined as separate fields to create an AND matching rule, instead of declaring within the same array field to create an OR rule. A new comment on a support ticket triggers the event.
The Step Functions Express Workflow
The application routes events to a Step Functions Express Workflow that is defined in the application’s SAM template:
FreshTracksZenDeskQueryMachine:
Type: "AWS::StepFunctions::StateMachine"
Properties:
StateMachineType: EXPRESS
DefinitionString: !Sub |
{
"Comment": "Create a new article from a zendeskTicket",
"StartAt": "GetFullZendeskTicket",
"States": {
"GetFullZendeskTicket": {
"Comment": "Get Full Ticket Details",
"Type": "Task",
"ResultPath": "$.FullTicket",
"Resource": "${GetFullZendeskTicket.Arn}",
"Next": "GetFullZendeskUser"
},
"GetFullZendeskUser": {
"Comment": "Get Full User Details",
"Type": "Task",
"ResultPath": "$.FullUser",
"Resource": "${GetFullZendeskUser.Arn}",
"Next": "PublishArticle"
},
"PublishArticle": {
"Comment": "Publish as an article",
"Type": "Task",
"Resource": "${CreateZendeskArticle.Arn}",
"End": true
}
}
}
RoleArn: !GetAtt [ MyStatesExecutionRole, Arn ]
This application is suited for a Step Functions Express Workflow because it is orchestrating short duration, high-volume, event-based workloads. Each workflow task is idempotent and stateless. The Express Workflow carries the workload’s state by passing the output of one task to the input of the next. The Amazon States Language ResultPath definition is used to control where each tasks output is appended to workflow’s state before it is passed to the next task.
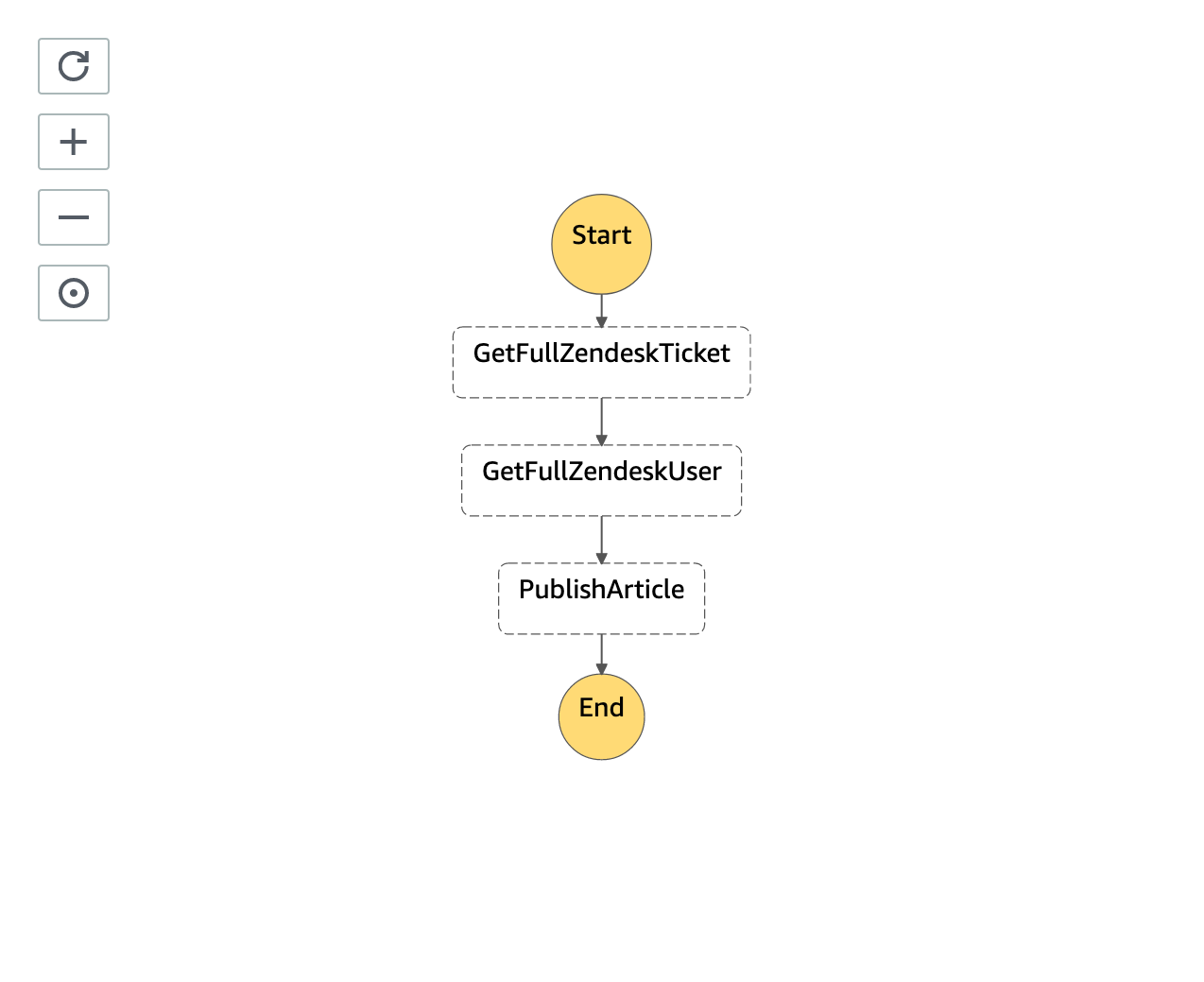
AWS StepFunctions Express workflow
Lambda functions
Each task in this Express Workflow invokes a Lambda function defined within the example application’s SAM template. The Lambda functions use the Node.js Axios package to make a request to Zendesk’s API. The Zendesk API credentials are stored in the Lambda function’s environment variables and accessible via ‘process.env’.
The first two Lambda functions in the workflow make a GET request to Zendesk. This retrieves additional data about the support ticket, the author, and the agent’s response.
The final Lambda function makes a POST request to Zendesk API. This creates and publishes a new article using this data. The permission_group and section defined in this function must be set to your Zendesk account’s default permission group ID and FAQ section ID.
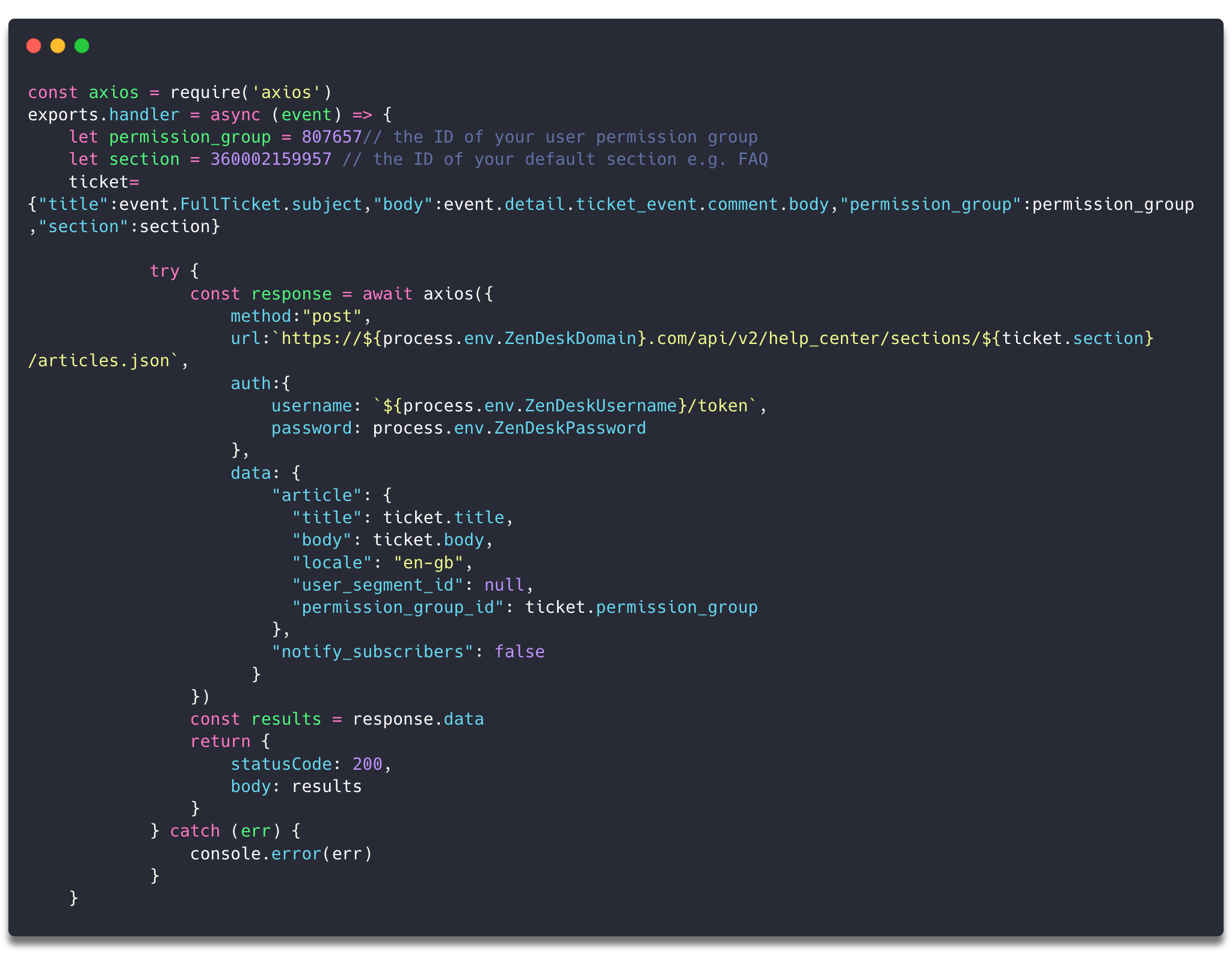
AWS Lambda function code
Integrating with your front-end application
Follow the instructions in the Fresh Tracks repo on GitHub to deploy the front-end application. This application includes Zendesk’s web widget script in the index.html page. The widget has been customized using Zendesk’s javascript API. This is implemented in the navigation component to insert custom forms into the widget and prefill the email address field for authenticated users. The backend application starts receiving Zendesk emitted events immediately.
The video below demonstrates the implementation from end to end.
Conclusion
This post explains how to set up EventBridge’s third-party integration with Zendesk to capture events. The example backend application demonstrates how to filter these events, and send downstream to a Step Functions Express Workflow. The Express Workflow orchestrates a series of stateless Lambda functions to gather additional data about the event. Zendesk’s API is then used to publish a new help guide article from this data.
This pattern provides a framework for bidirectional event orchestration between AWS services, custom web applications and third party integration partners. This can be replicated and applied to any number of third party integration partners.
This is implemented with minimal code to provide near real-time streaming of events and without adding latency to your application.
The possibilities are vast. I am excited to see how builders use this bidirectional serverless pattern to add even more value to their third party services.
Start here to learn about other SaaS integrations with Amazon EventBridge.