AWS Database Blog
Build a web-based cryptocurrency wallet tracker with Amazon Managed Blockchain Access and Query
From startups like digital asset wallet providers to enterprises like banks, companies are launching digital asset products that provide end users the ability to buy, sell, exchange and monitor their digital assets, such as cryptocurrency. Whether the end user is an institutional investor or a retail investor / enthusiast, all of these digital asset products require accessible user-experiences that display pertinent data related to a user’s digital assets. This data includes but is not limited to current and historical asset balances, historical transaction lists, transaction events, and more for each blockchain network on which assets are traded and owned.
To build such user interfaces for end users to view their digital asset balances on one or more public blockchains, one must invest in resource-intensive blockchain indexing infrastructure and complex extract transform and load (ETL) pipelines to serve data to frontend applications. However, these indexing and ETL resources are not heavily differentiated. Building these features in-house diverts resources from use-case specific functionality that differentiate a product from its competitors. The same case can be made for operating a fleet of public blockchain nodes to serve request traffic to send transactions to the Bitcoin blockchain, for example, which requires ongoing operating costs that can be shed in favor of API-driven solutions that bill only for requests made to a given blockchain.
With the launch of Amazon Managed Blockchain Access and Amazon Managed Blockchain Query, customers building digital asset applications and user experiences (UX) can trade the undifferentiated heavy lifting involved in blockchain node and data operations for on-demand, pay-as-you go services that serve the same requirements. This drastically reduces the complexity and number of AWS services required to accomplish the same functionality, and offers predictable costs based on usage of a set of APIs for blockchain data queries and JSON-RPC requests to public blockchains.
In this post, you learn how to use Amazon Managed Blockchain Query and Amazon Managed Blockchain Access, along with a handful of other AWS services to deploy a React frontend application that displays digital asset data from the Bitcoin and Ethereum blockchains in a matter of minutes. You can refer to the public code repository to use these React components in your own wallet, portfolio tracker, or other digital asset application.
AMB Access and Query drastically lower the barriers for customers to build quality user experiences with blockchain data. For more information, check out the solution guidance on building wallet solutions on the AWS Solutions Library.
The solution
To build an application that allows a user to view their digital asset balances on multiple blockchains, you will need to host a web application that is publicly accessible and can support users connecting their digital asset wallet to the application. In Figure 1, this architecture diagram illustrates the core AWS services and components that make up the digital asset portfolio tracker sample application.
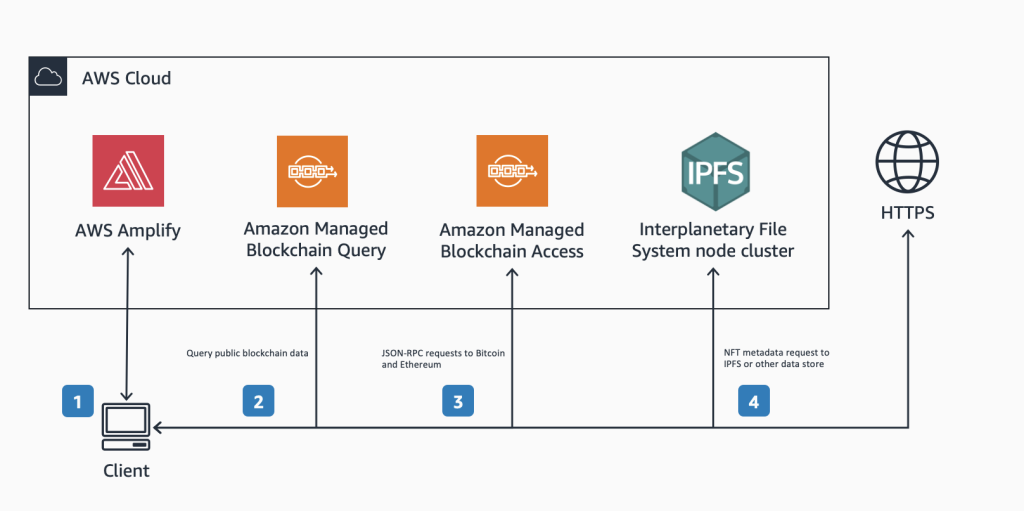
Figure 1
- AWS Amplify, a service that offers a complete set of tools to build, ship and host full-stack applications, serves the React web application via Hosted UI.
- To manage authentication with AWS services like Amazon Managed Blockchain using Signature Version 4 from a public frontend application, the application uses Amazon Cognito, which provides tools for identity storage using open identity standards. An Amazon Cognito Identity Pool is configured for guest access (unauthenticated identities) to allow users of the web app to use Amazon Managed Blockchain services without signing up for an account. Using the Amazon Cognito Identity Pool basic (classic) authflow, the web app makes
GetId
andGetOpenIdToken
requests to the Amazon Cognito API and receives an OAuth 2.0 token for each unauthenticated identity. The web app exchanges the OAuth 2.0 token for AWS API credentials by making anAssumeRoleWithWebIdentity
API request to the AWS Security Token Service (STS) API and providing the ARN of an AWS IAM Role that grants permission to Amazon Managed Blockchain. - Note that by enabling guest access with Amazon Cognito Identity Pools you opening up your Amazon Managed Blockchain services to the public. You could alternatively build authentication into your app and require users to create accounts and sign in to your application using Amazon Cognito User Pools.
- To manage authentication with AWS services like Amazon Managed Blockchain using Signature Version 4 from a public frontend application, the application uses Amazon Cognito, which provides tools for identity storage using open identity standards. An Amazon Cognito Identity Pool is configured for guest access (unauthenticated identities) to allow users of the web app to use Amazon Managed Blockchain services without signing up for an account. Using the Amazon Cognito Identity Pool basic (classic) authflow, the web app makes
- The web application makes requests to Amazon Managed Blockchain Query for token balances and transaction history on public blockchains such as Bitcoin and Ethereum. The majority of data used to fill user interface components for the digital asset portfolio tracker come from Amazon Managed Blockchain Query. Amazon Managed Blockchain Query APIs are designed to scale with your needs, allowing you to query public blockchain data at the volume that suits your workload without managing underlying blockchain infrastructure.
- The sample code provided in this blog uses the Amazon Managed Blockchain Query JavaScript SDK to make API requests to the following endpoints:
BatchGetTokenBalance
for Bitcoin balances of multiple addressesListTokenBalances
for ERC20 token balances of multiple externally owned accounts (EOA)ListTransactions
for lists of Bitcoin and Ethereum transaction hashesGetTransaction
for Bitcoin and Ethereum transaction detailsListTransactionEvents
for Bitcoin and Ethereum transaction events
- The Amazon Managed Blockchain Query API results can be paired with a third party API to get token prices and additional metadata. The sample code provided in this blog utilizes the CoinGecko Public API.
- The sample code provided in this blog uses the Amazon Managed Blockchain Query JavaScript SDK to make API requests to the following endpoints:
- For specialized requests that require access to a blockchain node, the web application can make JSON-RPC requests to a dedicated Ethereum full node or serverless Bitcoin JSON-RPC API endpoint on Amazon Managed Blockchain Access to interact with smart contracts, retrieve additional transaction metadata, broadcast transactions, and more. Amazon Managed Blockchain Access is not included in the sample code provided in this blog post, but can be extended to do so.
- Optionally, the web application can make requests for Non-Fungible Token (NFT) metadata (e.g. JSON attributes, images, videos) hosted on the web and/or the Interplanetary File System (IPFS) using an IPFS node cluster. IPFS is a protocol for storing and routing to files in a distributed network of nodes. If you would like to deploy your own IPFS cluster, you can refer to the AWS Solution Library Guidance for Deploying a Serverless IPFS Cluster on AWS. The sample code provided in this blog does not include NFT functionality, but can be extended to do so.
To deploy this architecture, with exception of Amazon Managed Blockchain Access and the IPFS cluster, you can use AWS Cloud Development Kit (CDK). The CDK is an infrastructure as code tool that allows you to model your applications in code and deploy them to AWS in an automated manner. In the next section, you will learn how to use the provided CDK code to deploy the web application using AWS Amplify as well as create an Amazon Cognito Identity Pool and AWS IAM Role to enable guest access to Amazon Managed Blockchain Query.
Deploy the AWS Resources using AWS Cloud Development Kit (CDK)
Prerequisites
- An AWS account and an IAM User
- AWS CLI installed and configured with user credentials
- Node.js to install project dependencies
Steps to deploy
After ensuring all pre-requisites are met, you can change into the code repository’s infrastructure directory, install dependencies, bootstrap your CDK environment, and run the deployment command:
This command deploys the following AWS resources:
- AWS CodeCommit repository to host source code and act as AWS Amplify App source
- Amazon Cognito Identity Pool and AWS IAM Roles to provide anonymous guest access to Amazon Managed Blockchain Query
- AWS Amplify App to build, deploy, and host the static React single page app. AWS CodeCommit repository is set as the app source to automatically deploy the app on commits to the main branch
During the deployment, the CDK will ask you to confirm IAM Statement changes. Review the changes and select y to continue as pictured below:
This process also prints a series of variable values to the console, including those which you will need in future steps (identified in red below):
This URL is where your application code will be committed:
This URL is where your frontend application will be hosted:
Building, deploying, and hosting the React web application
After deploying the core AWS resources, you must build and deploy the web application using AWS Amplify. The CDK app deployed in the prior step configured the Amplify App to automatically build, deploy, and host our web app on commits to the main branch of the CodeCommit repository. Follow the steps below to push the application source code to AWS CodeCommit:
- Authenticate your local git repository with AWS CodeCommit:
- The simplest way to do this is to generate Git credentials for CodeCommit for your IAM user in the IAM console then use those credentials for HTTPS connections to CodeCommit
- Be sure to save the credentials as they will be needed in the next steps when pushing to CodeCommit
- If you’d like to authenticate with CodeCommit using another mechanism such as federated access or temporary credentials, please see the CodeCommit documentation
- The simplest way to do this is to generate Git credentials for CodeCommit for your IAM user in the IAM console then use those credentials for HTTPS connections to CodeCommit
- Set the remote URL of your repo to the CodeCommit HTTPS Clone URL output by the
cdk deploy
command:- You should be prompted for the user name and password for the repository that you generated in Step 1 of this section. Depending on the configuration of your local computer, this prompt either originates from a credential management system for the operating system, a credential manager utility for your version of Git (for example, the Git Credential Manager included in Git for Windows), your IDE, or Git itself. Enter the user name and password generated for Git credentials
- After successful authentication, the repo will be pushed to AWS CodeCommit and will kickoff the Amplify App build and deploy, progress of the deployment can be monitored in the AWS Amplify Console
- When the Amplify deployment is complete, the web app will be live at the Amplify App URL output at the end of the
cdk deploy
command output
Once deployed, your portfolio tracker web application will be live at the URL returned by the CDK deployment in the first step. It will look something like this after a Bitcoin and/or Ethereum address is entered:
Cleanup steps
To avoid unwanted charges for AWS services, when complete, you may remove all resources deployed by AWS CDK using the stack removal command:
If you generated Git credentials for your IAM user, you can delete them from AWS IAM Console:
Conclusion
In this post, you learned about the value of managed services like Amazon Managed Blockchain that provide abstractions of common undifferentiated heavy lifting in blockchain / digital asset applications. By shedding the burden of node operations, blockchain data indexing, and ETL, you can focus your efforts on use-case specific functionality that moves the needle on adoption, such as quality user experience and data-driven insights in a portfolio tracker. To get hands-on with Amazon Managed Blockchain Query with a graphical user interface (GUI) to test its APIs, you can do so using the AWS Management Console Query Editor experience.
About the authors
Forrest Colyer manages the Web3/Blockchain Specialist Solutions Architecture team that supports the Amazon Managed Blockchain (AMB) service. Forrest and his team support customers at every stage of their adoption journey, from proof of concept to production, providing deep technical expertise and strategic guidance to help bring blockchain workloads to life. Through his experience with private blockchain solutions led by consortia and public blockchain use cases like NFTs and DeFi, Forrest helps enable customers to identify and implement high-impact blockchain solutions.
Ryan Canty is a Cloud Application Developer at AWS Professional Services. He enables enterprise cloud adoption by working directly with customers to realize their business outcomes and deliver cloud initiatives across all industries. With his background as an Application Engineer for an IoT startup, Ryan specializes in cloud native application architecture and development.